Converting string to array in JavaScript?
Converting a string to an array in JavaScript involves breaking down the string's characters into individual elements of an array. Here are a few approaches to achieve this:
Using split() method
The split() method splits a string into an array of substrings based on a specified delimiter. If no delimiter is provided, it treats the entire string as a single element.
Using spread operator
The spread operator can be used to transform a string into an array of characters directly.
Using Array.from()
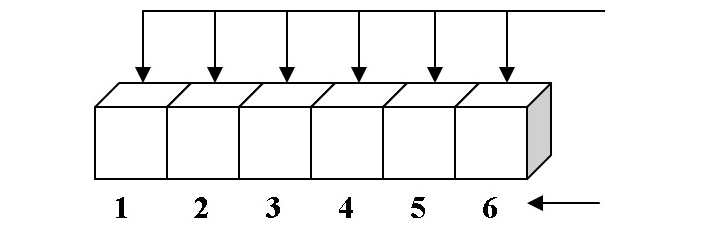
The Array.from() method can also convert a string into an array of characters.
Using split() with regular expression for words
To split a string into words, you can use a regular expression with the split() method.
Conclusion
Converting a string to an array in JavaScript involves various methods. The spread operator (...) or using the split() method with or without a regular expression are common approaches to transform a string into an array of individual characters or words, depending on the desired outcome.