Convert object to array in Javascript
The utility of Object.entries() has been accessible since the inception of ECMAScript 2017. This integral method facilitates the retrieval of an array comprising sub-arrays, each representing enumerable property key-value pairs that are directly situated on the object.
Object.keys
The Object.keys method operates by yielding a fresh array that carefully compiles all the keys of an object, organized in a coherent and sequential manner.
Object.values
The Object.values method facilitates the generation of a novel array encompassing all the values derived from an object, carefully arranged in a sequential and orderly fashion.
Array.prototype.map()
The attainment of an equivalent outcome can be realized through the strategic utilization of the map() function in conjunction with the Object.keys() method.
In the above code, the Object.keys() operation plays a key role by generating an array that encapsulates the enumerable string-based property names exclusive to the specified object. Subsequently, the Array.prototype.map() function comes into play, orchestrating the creation of a fresh array. This newly formed array is carefully populated with the outcomes derived from invoking a designated function on each individual element encompassed within the original array.
Destructuring Object.entries()
Destructuring serves as the mechanism that enables the extraction of values from arrays. In the provided code, the Object.entries() method yields subarrays, wherein each subarray comprises distinct key-value pairs, demarcated by a comma. To transform this hierarchical arrangement into a singular one-dimensional array, the process of destructuring becomes essential.
JavaScript Earlier Approach - Object to Array
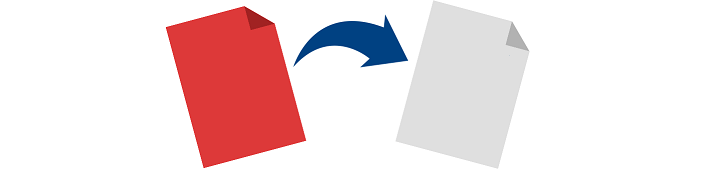
A precedent technique in JavaScript involved the iterative traversal of an object, with subsequent incorporation of individual object properties into a newly constructed array.
Conclusion
Converting objects to arrays in JavaScript can be achieved through methods like Object.entries(), which creates an array with subarrays holding key-value pairs, or by iterating through the object's properties and pushing them into a new array. These approaches facilitate the transformation of object data into a more structured array format.