How to Check Your Python Version?
To obtain the version of the currently running Python instance, you can utilize either the sys module or the platform module from the standard library. These modules offer a means to retrieve the precise Python version in use.
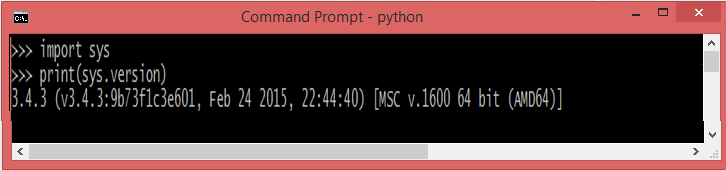
From command line
You can see which version of Python is installed on your OS using the command line .
- python -V
(Note: capital 'V')

Which version of Python is running my script?
In Python 2.7 and subsequent versions, the elements within sys.version_info can also be accessed using their respective names. For instance, sys.version_info[0] corresponds to sys.version_info.major, and this equivalence holds for other components as well.
Here, sys.version_info[0] is the major version number. sys.version_info[1] would give you the minor version number.
Platform Module in Python
Python encompasses a built-in module known as "platform," which furnishes comprehensive system information. Employing the platform module, one can effortlessly retrieve the version of the currently active Python instance.
Python undergoes regular updates incorporating new features and enhanced support. The evolution of Python versions spans from its inception in 1994 up to the present day. The Python Implementation commenced in December 1989, culminating in the release of version 1.0 in January 1994. Comparable to numerous programming languages, Python releases are organized chronologically by their respective release dates.
Python Versions
The standardized versioning scheme is devised to accommodate diverse identification methods across various Python projects, whether public or private. It involves a tuple that encompasses five key components of the version number: major, minor, micro, release level, and serial. While the majority of values are integers, the release level encompasses terms like 'alpha,' 'beta,' 'candidate,' or 'final.' For instance, the version_info value corresponding to Python version 2.0 is represented as (2, 0, 0, 'final', 0).
Run the Python command-line interpreter:
- Open Command line: Start menu -> Run and type cmd
- Type: C:\Python34\python.exe
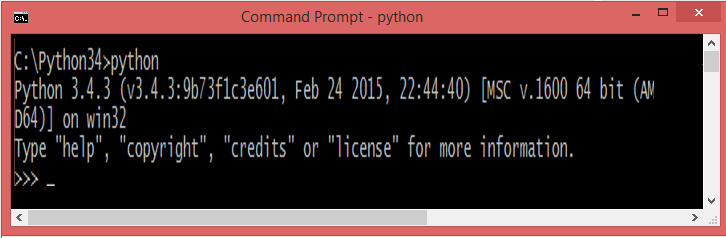
Here you can see, Python version is displayed on console immediately as you start interpreter from command line. The details are a string containing the version number of the Python interpreter plus additional information on the build number and compiler used.
From command line
You can check which version of Python is installed on your operating system using the command line using the following command.
- C:\Python34> Python -c "print(__import__('sys').version)"

Check Python Version from your program
You can programmatically determine which version of Python is installed on the system where the Python script is running.
For example, to check that you are running Python 3.x , use:
Conclusion
To determine the Python version in use, you can utilize the sys module's version attribute or the platform module, which offers comprehensive system information. Both methods provide insights into the version number and other relevant details, enabling you to ensure compatibility and make informed decisions.