FileWriter Class in Java
The FileWriter class in Java is used to write characters to a file. It provides a convenient way to write character data to a file as a stream of characters. FileWriter extends the OutputStreamWriter class, which means it writes characters to an output stream, and in the case of FileWriter, it writes them to a file.
To use the FileWriter class, you need to create an instance of it and specify the file path that you want to write to. You can then use various methods provided by the FileWriter class to write characters to the file.
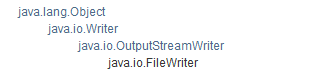
Follwoing is an example of how to use the FileWriter class to write data to a file:
In this example, we create a FileWriter object with the file path "output.txt". We then use the write() method of the FileWriter class to write three lines of text to the file. Each call to the write() method appends the data to the end of the file.
After running this code, a new file named "output.txt" will be created in the same directory as the Java program, and the three lines of text will be written to the file.
File already exists
It's important to note that when using the FileWriter class, the file is automatically created if it does not exist. If the file already exists, the FileWriter will overwrite the existing content. To append data to an existing file without overwriting it, you can use the FileWriter constructor with a second boolean parameter set to true.
This will create a FileWriter that appends data to the existing file instead of overwriting it.
Java FileWriter with append mode
FileWriter class allows you to open a file in "append mode," which means you can add data to an existing file without overwriting its contents. To use FileWriter in append mode, you need to pass true as the second parameter to the constructor.
Follwoing is an example of how to use FileWriter with append mode:
In this example, we open the file "data.txt" in append mode by passing true as the second parameter to the FileWriter constructor. The three lines of text will be added to the end of the existing file if it already exists. If the file doesn't exist, it will be created.
After running this code, if "data.txt" already existed and contained some data, the three new lines will be appended to the existing content. If "data.txt" did not exist before, it will be created with the three lines as its content.
Appending data to an existing file is useful when you want to add new information to a file without losing the previous data. It's commonly used in log files, where new log entries are added to the file as events occur.
Remember to handle any potential IOException that may occur during file writing, as shown in the example above. Also, ensure that you close the FileWriter after you finish writing to release system resources properly. The try-with-resources statement used in the example automatically closes the FileWriter when the try block is exited.
Conclusion
The FileWriter class in Java provides a straightforward way to write character data to a file. It's essential to handle any potential IOExceptions that may occur during file writing operations. Additionally, if you need more advanced writing capabilities, consider using BufferedWriter or PrintWriter, which provide additional buffering and formatting options.