Get User Input from Keyboard - input() function | Python
To gather input from the keyboard, Python employs the built-in function input(). This function features an optional parameter, often referred to as "prompt," which is a string displayed on the screen whenever the function is invoked.
Syntax- prompt: A String, representing a default message before the input.
The input() function prompts for text input when provided with a parameter. This function reads input from the keyboard, converting it into a string format while eliminating the newline (Enter) character. It's advisable to provide informative instructions to guide users in entering the anticipated values.
example
New line for input() in Python
When you run this program, the python ask the data to enter the same line. If you want to receive data on next line , put a "\n" inside of the quotes.
"\n" is a control character, sort of like a key on the keyboard that you cannot press.
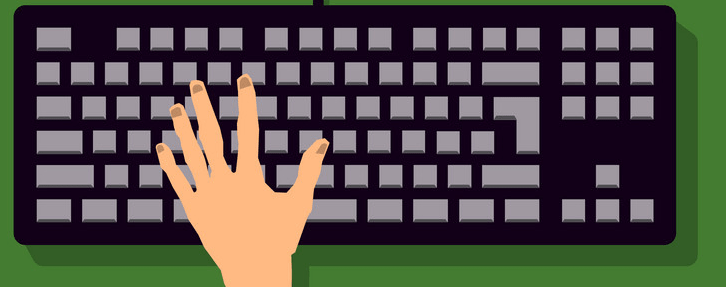
Python Integer as the User Input
To capture the input within your program, you'll require a variable, which serves as a container to store data. By default, the input() function converts all received information into a string. Consequently, obtaining an integer or other data types directly from user input is not feasible. However, built-in functions can be employed to convert the entered string into an integer or other desired types.
Conclusion
Acquiring user input in Python involves utilizing the built-in input() function, which captures keyboard input as a string by default. To retrieve specific data types like integers from user input, built-in conversion functions can be employed. This process involves using variables as containers to store the collected data.