ADO.NET Architecture
ADO.NET encompasses a comprehensive collection of Objects that effectively expose data access services within the .NET environment. As a prominent data access technology within Microsoft's .NET Framework, it bridges the communication gap between relational and non-relational systems by utilizing a standardized set of components.
At the heart of ADO.NET lies the System.Data namespace, which serves as the foundation for all data providers. This namespace houses a plethora of classes that are utilized by various data providers, ensuring a cohesive and consistent experience across the board. By adhering to this shared namespace, ADO.NET maintains a unified approach to data access.
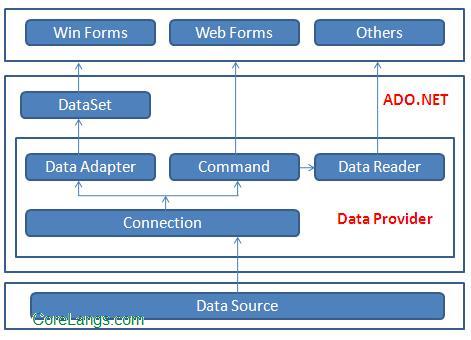
ADO.NET is designed with user-friendliness in mind, offering an intuitive experience for developers. In this regard, Visual Studio, a popular integrated development environment, further simplifies the process by providing numerous wizards and additional features. These tools allow developers to effortlessly generate ADO.NET data access code, streamlining the development process and enhancing productivity.
Data Providers and DataSet
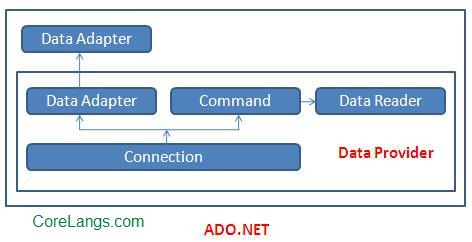
ADO.NET revolves around two fundamental components: Data Providers and DataSet. These components play crucial roles in facilitating efficient data management within the framework.
Data Providers are a collection of classes specifically designed to interact with various types of data sources. These classes serve as the intermediaries between the application and the underlying databases, enabling seamless execution of data management operations. Whether it is retrieving data, performing updates, or executing queries, Data Providers handle the intricate tasks associated with specific databases, ensuring smooth and efficient data access.
On the other hand, the DataSet class acts as a powerful mechanism for managing data when it is disconnected from the original data source. It serves as a container that holds data retrieved from a database and allows for flexible manipulation and management of this data within the application. By operating independently from the data source, the DataSet class empowers developers to work with data in an offline or disconnected state, facilitating efficient data synchronization and manipulation.
Together, the Data Providers and DataSet form a cohesive framework within ADO.NET, providing developers with the necessary tools and mechanisms to interact with and manage data effectively. While the Data Providers establish connections and perform operations on specific databases, the DataSet class ensures seamless management and manipulation of data, even when disconnected from the original data source.ce.
Data Providers
Within the .NET Framework, there are primarily three Data Providers available for ADO.NET: the Microsoft SQL Server Data Provider, the OLEDB Data Provider, and the ODBC Data Provider. Each of these providers is specifically tailored to work with different types of databases and utilizes distinct connection objects for establishing communication with the respective database systems.
The Microsoft SQL Server Data Provider is specifically designed for interacting with Microsoft SQL Server databases. To establish a connection with SQL Server, developers employ the SqlConnection object provided by this Data Provider. This connection object serves as the gateway for accessing and manipulating data within SQL Server databases.
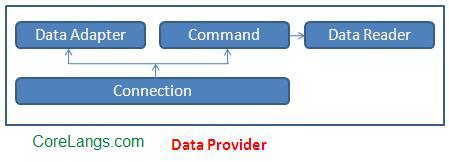
The OLEDB Data Provider, on the other hand, facilitates connectivity with databases that support the OLEDB (Object Linking and Embedding, Database) technology. To establish a connection with OLEDB-compliant databases, such as Oracle or Access, developers utilize the OleDbConnection object. This object acts as the conduit for interacting with the OLEDB-supported databases, enabling efficient data access and manipulation.
Lastly, the ODBC Data Provider is designed to work with databases that conform to the ODBC (Open Database Connectivity) standard. It offers a universal approach to database connectivity, enabling interaction with a wide range of database systems. The OdbcConnection object is used to establish connections with ODBC-compliant databases, allowing seamless data access and management.
Connection
The Connection Object within ADO.NET serves as the means to establish a physical connection to the data source. In order to establish this connection successfully, the Connection Object requires specific information that allows it to identify and authenticate with the data source. This crucial information is typically provided through a connection string.
A connection string is a string of parameters that contains the necessary details for establishing a connection with the data source. It includes information such as the data source location (e.g., server name or file path), credentials (e.g., username and password), and any other required settings specific to the data source.
Command
The Command Object is a vital component in ADO.NET that facilitates the execution of SQL statements or stored procedures at the data source. It serves as the bridge between the application and the data source, allowing developers to interact with the data by issuing SQL queries or executing stored procedures.
To execute SQL statements or stored procedures, the Command Object provides a range of Execute methods. These methods offer different ways to perform the SQL queries, providing flexibility and versatility to developers. Depending on the specific requirements of the application, developers can choose the most suitable Execute method to execute the desired SQL command.
The Execute methods offered by the Command Object allow for various execution scenarios. They can be used to retrieve results from the data source, modify data within the data source, or execute non-query commands that do not return any data. The choice of the appropriate Execute method depends on the type of SQL command being executed and the expected outcome.
DataReader
The DataReader Object plays a crucial role in ADO.NET by providing a stream-based, forward-only mechanism for retrieving query results from the data source. Unlike other components, the DataReader is specifically designed for read-only operations and does not support data modification.
One key characteristic of the DataReader is its stream-based nature. This means that it retrieves data from the data source in a sequential and forward-only manner. It reads the data row by row, allowing the application to process and consume the data in a sequential fashion.
Additionally, the DataReader is optimized for performance and memory efficiency. It efficiently streams data from the data source as it is being read, eliminating the need to load the entire result set into memory. This makes it highly suitable for working with large datasets where memory consumption is a concern.
DataAdapter
The DataAdapter Object plays a critical role in ADO.NET by facilitating the population of a Dataset Object with results retrieved from a data source. It acts as a bridge between disconnected Dataset objects and the physical data source, enabling seamless interaction and synchronization between the two.
When working with ADO.NET, the Dataset Object serves as an in-memory representation of data retrieved from a data source. It provides a disconnected and independent storage for the data, allowing for efficient manipulation and processing within the application. However, to initially populate the Dataset Object with data, the DataAdapter Object comes into play.
The DataAdapter's primary purpose is to fetch data from the data source and populate the Dataset Object with the retrieved results. It handles the communication and data retrieval process, utilizing the appropriate Connection Object to establish a connection with the data source. Once the connection is established, the DataAdapter executes the necessary SQL statements or stored procedures and retrieves the corresponding data.
Upon retrieving the data, the DataAdapter maps the results to the structure of the Dataset Object. It populates the Dataset's tables, rows, and columns with the retrieved data, ensuring that the in-memory representation aligns with the data structure from the data source.
DataSet
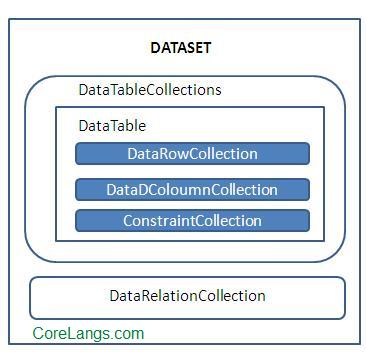
The DataSet Object in ADO.NET serves as a disconnected representation of result sets retrieved from a data source. It provides a flexible and independent in-memory storage for data, enabling efficient manipulation and management within the application.
Being disconnected means that the DataSet is not directly connected to the data source. Once the data is retrieved and stored in the DataSet, the connection to the data source is no longer required. This disconnected nature allows for greater flexibility and mobility in working with the data, as the application can manipulate and process the data without the need for a constant connection to the data source.
The DataSet is entirely independent from the data source. It acts as a self-contained entity, holding its own representation of data with its own internal structure. This independence allows the DataSet to be used in various scenarios, such as offline processing, data caching, or transferring data between different components of an application.
Within the DataSet, data is organized into rows and columns, similar to a traditional database table. It also supports defining primary keys, constraints, and relationships between DataTable objects. This means that multiple DataTable objects within the DataSet can be related to each other using DataRelation objects, providing a powerful mechanism for managing complex data relationships.
To bridge the gap between the DataSet and the data source, the DataAdapter Object comes into play. The DataAdapter acts as an intermediary, facilitating communication and synchronization between the DataSet and the data source. It provides methods to populate the DataSet with