Email Address Validation
An email address is a string comprising a subset of ASCII characters that is divided into two parts by the "@" symbol. The section preceding the "@" sign is known as the local part of the address, while the portion following the "@" sign represents the domain name to which the email message will be directed. In the provided example, "feedback" is the local part, and "net-informations.com" is the domain name.
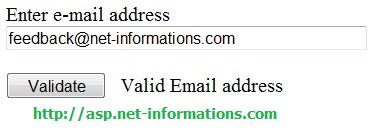
To ensure the validity of email addresses, we can employ a regular expression pattern for validation purposes. Regular expressions consist of patterns that describe specific text sequences. In the .NET Framework, regular expression classes are part of the base class library and can be utilized in various programming languages and tools targeting the common language runtime, including ASP.NET and Visual Studio .NET.
Regular expressions
One useful method for working with regular expressions is the Regex.IsMatch method, which determines whether a given input string matches a specified regular expression pattern. The pattern parameter incorporates different elements of the regular expression language, symbolically defining the pattern to be matched within the string.
To illustrate the implementation of email address validation using regular expressions in an ASP.NET program, the following code snippet can be employed:
The pattern parameter consists of various regular expression language elements that symbolically describe the string to match pattern . The following ASP.NET program shows how to validate an email address with the help of regular expressions.
Default.aspxConclusion
Using regular expressions, developers can effectively validate the format and structure of email addresses, ensuring that they adhere to the expected pattern and minimizing the risk of accepting incorrect or malformed email addresses within their applications.