ASP.NET File Upload
The FileUpload server control provided by ASP.NET is a highly capable tool that offers a convenient solution for a task that was considerably challenging in the earlier days of Active Server Pages 3.0. This control empowers end-users to effortlessly upload one or multiple files to the server hosting your application. It serves as a powerful mechanism for accepting file submissions from users, facilitating seamless data exchange.
FileUpload control
Using the FileUpload control, you can easily manage the size of the uploaded files. By default, ASP.NET allows a maximum file size of 4MB. However, if you need to handle larger files, you have the flexibility to adjust the size limit by modifying settings in either the web.config.comments or web.config file. This capability ensures that your application can accommodate varying file sizes based on your specific requirements.
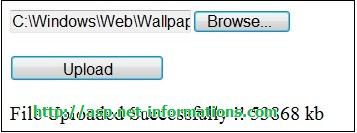
To incorporate the FileUpload control into your application, simply drag and drop the control onto the desired form. Once placed, you can use the control by writing the necessary code within the upload button's click event. This event handler enables you to define the logic and actions to be executed when the user initiates the file upload process.
Moreover, the FileUpload control offers the capability to specify the location where the uploaded file should be saved on the server. This feature allows you to define a specific directory or path to store the uploaded files, ensuring proper organization and easy retrieval of the submitted data.
VB.NetConclusion
The FileUpload server control in ASP.NET provides a powerful and user-friendly solution for enabling file uploads within your web application. With its drag-and-drop functionality and straightforward event handling, developers can effortlessly incorporate file upload functionality into their forms. The control's flexibility regarding file size and the ability to specify the upload destination further enhances its utility, allowing for seamless integration and management of file submissions.