Python Fundamentals
Keywords in Python
In Python programming, Keywords hold a special status as they are reserved words with predefined meanings, critical to the language's functionality. These exclusive words cannot be repurposed as names for any entities, including variables, classes, or functions within the Python codebase. This design choice ensures the language's consistency and prevents ambiguity during execution.
To explore the comprehensive roster of Python Keywords, developers can readily access the list through the Python console's help utility. Familiarizing oneself with these reserved words not only helps in steering clear of naming conflicts but also enhances code readability and adherence to Python's standardized syntax. Embracing this fundamental aspect of Python's structure empowers developers to utilize the full potential of the language and craft elegant, efficient, and maintainable code that adheres to best practices and coding conventions.
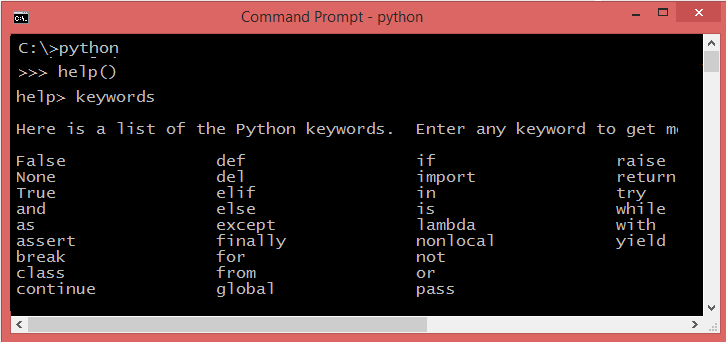
Python Variables and Data Types
A variable assumes the role of a versatile and fundamental construct, acting as a container to store values of different data types. By assigning a name to a variable, developers can conveniently reference and manipulate the associated value throughout the code. The Python interpreter, equipped with dynamic typing, dynamically deduces the data type of the variable based on the value assigned to it, facilitating seamless data handling and enabling developers to focus on the logic rather than the explicit data type declaration.
The case sensitivity of Python lends precision to the language, making variable names distinct and unambiguous, thereby upholding a clear distinction between uppercase and lowercase characters. In Python, variable names are carefully chosen to be descriptive, concise, and follow a specific set of rules. They must consist of alphanumeric characters (A-z, 0-9) and can begin with either a letter or an underscore (A-z, _). Adhering to these naming conventions ensures the conformity of the code to Python's standardized syntax and boosts code readability, thus maintaining a uniform and coherent coding practice.
Every value in Python has a datatype. Python supports the following data types:
- Numbers
- String
- List
- Tuple
- Dictionary
More on.... Python Variables and Data Types
Python Operators
Python Operators are special symbols that designate some sort of computation should be performed. Python includes operators in the following categories:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Boolean Operators
- Bitwise Operators
- Membership Operators
- Identity operators
More on.... Python Operators
Python Datatype Conversion
Python defines type conversion functions to directly convert one data type to another which is useful for program development.
- int() : float, string to integer
- float() : integer, string to float
- str(): integer, float, List, Tuple to string
- list() : string, Tuple, Dictionary to List
- tuple() : string , list to tuple
More on... Python Datatype conversion
Python Mathematical Function
the Python math module serves as a valuable resource, empowering programmers to utilize an extensive repertoire of common and essential mathematical operations seamlessly within their programs. This indispensable module opens the gateway to a plethora of mathematical functionalities, including addition (+), subtraction (-), division (/), and multiplication (*), all of which are crucial building blocks for countless computational tasks. Whether it's performing elementary arithmetic calculations or executing complex mathematical operations, the math module provides a comprehensive toolkit for precise and accurate results. Its wide-ranging capabilities transcend basic arithmetic, encompassing functions for trigonometry, logarithms, exponents, square roots, and much more.
Conclusion
By integrating the math module into their programs, developers elevate the sophistication and versatility of their code, streamlining data processing, numerical analysis, and scientific computing with utmost efficiency and precision. With Python's math module at their disposal, programmers can confidently embark on a myriad of mathematical explorations, enabling them to unlock the full potential of their programming endeavors with confidence and ease.