Type Conversion in Python
In Python, type conversion (also known as type casting) refers to the process of converting one data type into another. Python provides built-in functions to perform type conversion, allowing you to convert variables or values from one data type to another as needed.
There are two types of type conversion in Python:
- Implicit type conversion: This type of conversion is performed automatically by the Python interpreter. For example, if you add an integer and a string, the Python interpreter will automatically convert the string to an integer before performing the addition.
- Explicit type conversion: This type of conversion is performed by the user using the built-in type conversion functions. For example, the int() function can be used to convert a string to an integer.
Here are some common type conversion functions along with examples:
int() function
Converts a value to an integer data type.
float() function
Converts a value to a floating-point data type.
str() function
Converts a value to a string data type.
bool() function
Converts a value to a Boolean data type.
list(), tuple(), set() functions
Convert a sequence or iterable to a list, tuple, or set, respectively.
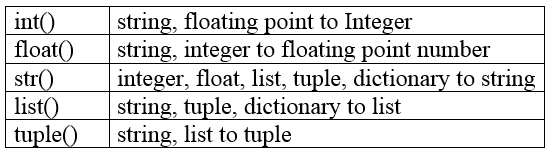
Conclusion
Type conversion is essential when you need to perform operations or utilize variables of different data types. It allows you to manipulate and combine data of various types, enhancing the flexibility and versatility of your Python programs. However, be cautious when converting data types, as some conversions may lead to loss of information or unexpected results.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples