Boxing and Unboxing in Java
Autoboxing and unboxing are two essential features included in Java 1.5. These features play a crucial role in simplifying the conversion process between primitive types and their corresponding wrapper classes. Autoboxing enables the automatic conversion from a primitive type to its corresponding wrapper class type, while unboxing facilitates the vice-versa conversion. This functionality significantly enhances the convenience and flexibility of programming in Java, as it eliminates the need for manual conversion code and reduces potential errors. By seamlessly handling the conversion between primitive types and wrapper classes, autoboxing and unboxing contribute to the overall efficiency and readability of Java code.
Java Boxing
Autoboxing is a fundamental process in programming where a primitive type data is automatically converted into an instance of its corresponding wrapper class. This process entails dynamic memory allocation and initialization of an object for each primitive value. The noteworthy aspect of autoboxing is that it eliminates the necessity of explicitly constructing an object, as the conversion is seamlessly handled by the programming language. By automating this process, autoboxing simplifies code implementation and enhances readability, enabling developers to focus on the essential logic of their programs rather than the intricacies of object creation.
Java Unboxing
Unboxing refers to the process of converting a wrapper instance into its corresponding primitive type. It involves automatically extracting the value from a boxed object type when its value is needed. Notably, this conversion occurs without the requirement of invoking specific methods like doubleValue() or intValue(). Instead, the unboxing process seamlessly retrieves the underlying primitive value, allowing for direct usage in computations or assignments. By eliminating the need for explicit method calls, unboxing enhances code readability and simplifies programming tasks, ultimately contributing to more efficient and concise code implementation.
example:When it required ?
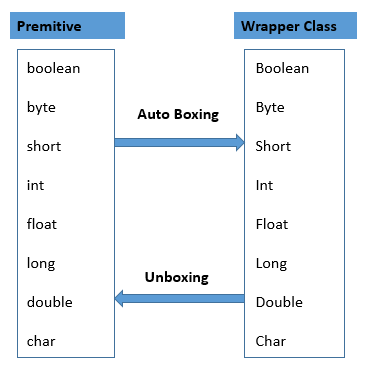
When a method is expecting a wrapper class object but the value that is passed as parameter is a primitive type. When primitive value assigned to wrapper class variable or reverse.
Advantages
No need to make object explicitly and wrap the value of primitive type.
Disadvantage
“Autoboxing” is too unexpected in its behavior and can easily result in difficult to recognize errors.
Performance
Autoboxing does create objects which is not clearly visible in the code. So when autoboxing occurs performance suffers.
What is a Wrapper Class ?
A wrapper class is a class that encapsulates or "wraps" the functionality of another class or component. Specifically, it wraps around a primitive data type and gives it the appearance and capabilities of an object. This allows the primitive data type to be used in situations where an object is required.
The primary advantage of using a wrapper class is that it enables the utilization of primitive data types as objects. By wrapping a primitive type, such as an integer or boolean, in a corresponding wrapper class (e.g., Integer or Boolean), it becomes possible to use the wide range of functions and operations available to objects. These wrapper classes provide methods to manipulate and perform various operations on the underlying primitive values, enhancing the flexibility and functionality of the programming language.
Conclusion
Boxing and unboxing in Java refer to the process of converting between primitive data types and their corresponding wrapper classes. Boxing is the process of converting a primitive type to its equivalent wrapper class, while unboxing is the process of extracting the value from a wrapper class and converting it back to its corresponding primitive type. These conversions are automatically handled by the Java compiler, making it convenient for developers to work with both primitive types and objects interchangeably.