Java Access Modifiers
Access modifiers are keywords in object-oriented programming languages that determine the visibility and accessibility of class members (e.g. fields, methods, constructors, etc.). They control which parts of the code can access these members and are used to enforce encapsulation, one of the fundamental principles of OOP. Different programming languages may have different access modifiers and different rules for their use, but the basic concept remains the same: to control the visibility and accessibility of class members.
Why are Access Modifiers crucial?
Access specifiers are important because they enforce encapsulation, one of the fundamental principles of object-oriented programming (OOP). Encapsulation helps to maintain the integrity of an object's state by hiding its implementation details and exposing only those parts of the object that are intended for public use.
By using access specifiers, you can control the visibility and accessibility of class members and prevent unwanted access or modification of internal data. This makes your code more robust, maintainable, and secure.
In addition, access specifiers can also help to promote modularity and reusability by allowing you to create classes that have a well-defined interface and can be easily integrated into other parts of your code.
Access modifiers in java
In Java, access modifiers are keywords used to control the visibility and accessibility of class members (e.g. fields, methods, constructors, etc.). Java has four access modifiers:
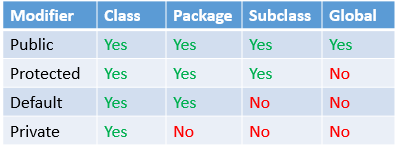
- Public: Members declared as public can be accessed from anywhere in the code.
- Protected: Members declared as protected can be accessed within the same class and its subclasses.
- Default (Package-private) : Members declared with no modifier (also called default or package-private visibility) can be accessed within the same package, but not outside of it.
- Private: Members declared as private can only be accessed within the same class.
Public Access Modifier
The "public" access modifier in Java is the most permissive access level. A member (i.e. class, method, variable) declared as public can be accessed from anywhere in the Java program.
Here, the class xClass and its method msg() and variable x are declared as public, which means they can be accessed from any other class in the Java program.
Protected Access Modifier
The protected access modifier in Java allows access to the members (i.e. classes, methods, variables) within the class in which they are declared and its subclasses. Members declared as protected can be accessed from within the same package or from a different package if they are subclasses of the class in which they are declared.
Here, the variable x and method msg() are declared as protected, which means they can be accessed within the xClass class and any of its subclasses. If a subclass is in a different package, it can still access the protected members. However, any class outside of the package and not a subclass of xClass will not be able to access the protected members.
Package-private Access Modifier (Default) or (no modifier)
In Java, when no access modifier is specified for a member (i.e. class, method, variable), it is given a default access level of "package-private" . Members declared with package-private access can only be accessed from within the same package.
In Java, when no access modifier is specified for a member (i.e. class, method, variable), it is given a default access level of "package-private" (Default) . Members declared with package-private access can only be accessed from within the same package.
Here, the variable x and method msg() have no explicit access modifier, so they are given the default package-private access level. This means they can only be accessed within the same package as the xClass class and cannot be accessed from any class in a different package.
Private Access Modifier
The private access modifier in Java is the most restrictive access level, allowing access to the members (i.e. classes, methods, variables) only within the class in which they are declared. Members declared as private cannot be accessed from any other class, even if it's in the same package.
Here, the variable x and method msg() are declared as private, which means they can only be accessed within the xClass class and cannot be accessed from any other class in the program.
What are Non-Access Modifiers in Java
Non-access modifiers are keywords that provide additional information about the properties and behavior of classes, methods, and variables. Non-access modifiers do not affect the visibility or accessibility of the members, but they provide additional information to the compiler, such as the intended behavior of the members.
Some of the most commonly used non-access modifiers in Java are:
- final: A final member cannot be overridden or modified.
- synchronized: A synchronized method is used for thread synchronization.
- transient: A transient variable is not part of the serialization process.
- static: A static member belongs to the class, rather than an instance of the class.
- abstract: An abstract member is a declaration only and does not have an implementation.
- volatile: A volatile variable is used to indicate that its value may be changed by multiple threads.
Here, MAX_COUNT is declared as final and static, meaning it cannot be changed and belongs to the class, rather than an instance of the class. abstractMethod is declared as abstract, meaning it is only a declaration and has no implementation. synchronizedMethod is declared as synchronized, meaning it is used for thread synchronization.
How Access Control and Inheritance in Java
When a subclass inherits from a superclass, it can access the public and protected members of the superclass. However, private members of the superclass are not accessible from the subclass.
Example:Here, the xSubClass subclass inherits from the xSuperClass superclass. The xSubClass class can access the x variable and msg() method from the xSuperClass superclass because they are declared as protected.
Which access Modifiers are not used for the class in Java
The private and protected access modifiers are not used for classes. Classes can only be declared as public or with no access modifier (which defaults to package-private).
The private access modifier is used to limit the visibility and accessibility of class members, such as methods and variables, to within the same class. The protected access modifier is used to allow access to class members from within the same class and its subclasses.
Since classes themselves cannot be subclasses, there is no need to use the protected access modifier for classes. The private access modifier also does not apply to classes because it is used to limit visibility and accessibility of class members, not the class itself.
Java Access Modifiers with Method Overriding
When a subclass overrides a method from a superclass, the visibility of the overridden method must not be less restrictive than the visibility of the method in the superclass.
Here, the xSubClass subclass overrides the msg() method from the xSuperClass superclass. The msg() method in xSuperClass is declared with the public access modifier, which allows it to be accessed from anywhere. The msg() method in xSubClass is also declared with the public access modifier, which is not less restrictive than the visibility of the method in xSuperClass.
If the msg() method in xSubClass were declared with a more restrictive access modifier, such as private or protected, a compile-time error would occur because the visibility of the overridden method in the subclass must not be less restrictive than the visibility of the method in the superclass.
Conclusion
Java access modifiers are keywords that determine the visibility and accessibility of classes, variables, methods, and constructors in Java programs. There are four main access modifiers in Java: public, private, protected, and default (package-private), each with different levels of access control to balance encapsulation and accessibility in object-oriented programming.