Python Dictionary
In Python, a dictionary is an unordered collection of key-value pairs, where each key is unique and associated with a corresponding value. Dictionaries are enclosed in curly braces {} and consist of comma-separated key-value pairs.
How to create a Python dictionary?
A Python dictionary can be created using curly braces, with each key-value pair separated by a colon. For example, the following code creates a dictionary called dict1 with two key-value pairs:
How to access a value in a Python dictionary?
To access a value in a Python dictionary, you can use the key. For example, the following code prints the value associated with the key key1 in the dictionary dict1:
Adding and updating entries in a dictionary
Removing entries from a dictionary
How to iterate through a Python dictionary?
You can iterate through a Python dictionary using a for loop. The for loop will iterate over the keys in the dictionary, and you can use the key to access the value associated with the key. For example, the following code iterates through the dictionary dict1 and prints the key and value for each pair:
Checking if a key exists in the dictionary
Why use Python dictionaries?
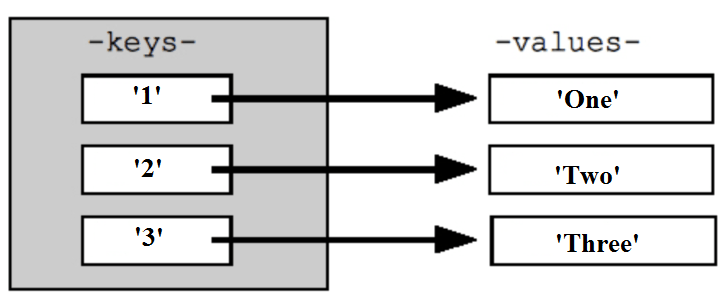
Python dictionaries are a powerful data structure that can be used to store a variety of data. They are especially useful for storing data that is associated with a unique key, such as the names and ages of people, or the inventory of a store. Dictionaries are also mutable, which means that they can be changed after they are created. This makes them a flexible data structure that can be used to store data that changes over time.
Conclusion
Dictionaries are versatile and widely used in Python for various tasks, such as storing and accessing data, mapping relationships, and processing key-value data efficiently. The ability to associate unique keys with corresponding values makes dictionaries a powerful data structure in Python.