How to Python list sort()
The sort() method in Python is employed to arrange the elements of a list in ascending, descending, or custom-defined order, thereby enabling efficient organization of the data. Upon invoking the sort() method without any arguments, it iterates over the list elements in a loop, effectively rearranging them in ascending order. To sort the list in descending order, one can utilize the reverse=True argument, which instructs the method to rearrange the elements in reverse order, thereby achieving a descending sequence.
sort() method
Python provides an elegant and straightforward way to arrange the elements of a list in the desired order, catering to the specific needs of data processing and analysis. The ability to customize the sorting order empowers programmers to handle diverse scenarios and optimize the organization of data for efficient retrieval and manipulation.
Syntax- reverse - Default is reverse=False, while reverse=True will sort the list descending.(Optional)
- key - A method to specify the sorting criteria (Optional)
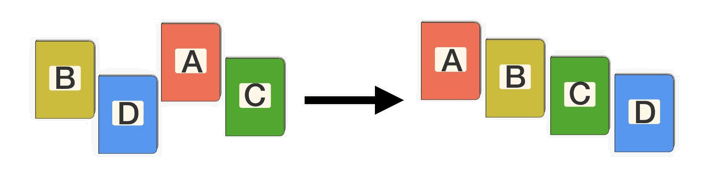
Sort list of numbers
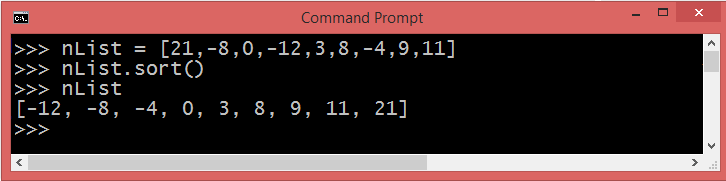
Sort list of strings
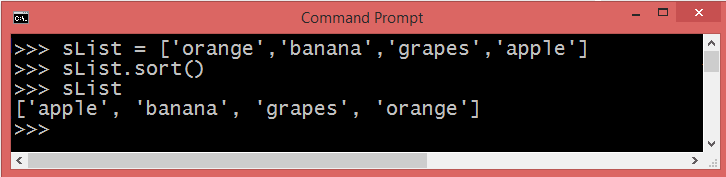
Sort list of strings in descending order
If any of the list element is Uppercase , let's see what happens.
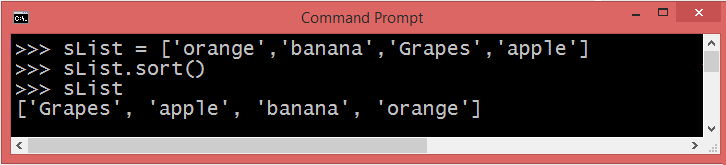
It is because Python treats all Uppercase letters to be lower than Lowercase letters, if you want you can change it.
key=str.lower
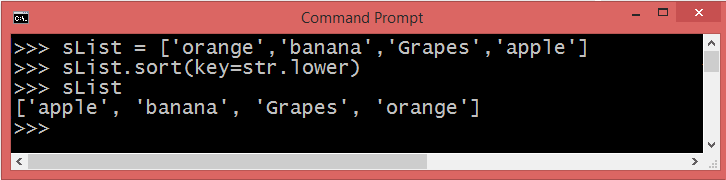
Sort using key parameter
The key parameter in the sort() method of a list allows you to specify a custom function that will be called on each item of the list before making comparisons during the sorting process. This parameter provides a way to define your own implementation for sorting the elements based on a specific criterion or attribute.
The key parameter is optional, and if provided, it should be a function that takes an item from the list as an argument and returns a value that will be used for sorting. The sort() method will use these generated values for comparison instead of the original items in the list.
Sort using key parameter (reverse)
Sort the list by the length of the values
A new function "sortLen" that returns the length of the list elements and add this function as key of sort() function.
Sorting a List of Tuples
A tuple is a collection of Python objects written within round brackets () and separated by commas. The key distinction between tuples and lists is that tuples are immutable, meaning their elements cannot be changed, added, or removed after the tuple is created. In contrast, lists are mutable, allowing modifications to their elements.
This immutability makes tuples suitable for situations where you need fixed data or want to ensure the integrity of the data throughout your program's execution. In contrast, lists are more appropriate when you require a data structure that can be modified, expanded, or contracted during the course of your program.
Sorting a List of Tuples by the first item
Sorting a List of Tuples by the second item
Sorting a list of objects
Conclusion
The sort() method is used to sort the elements of a list in ascending order. It rearranges the elements in place, directly modifying the original list without creating a new one. Additionally, the sort() method allows for custom sorting by using the key parameter, which specifies a function to be applied to each element before making comparisons.