Merging two Dictionaries | Python
The primary operations on a Python dictionary involve storing values with corresponding keys and retrieving values using the keys. In Python, there are multiple techniques to merge two dictionaries, and this article explores various approaches to accomplish this task efficiently.
Although Python lacks a built-in function for concatenating or merging two dictionaries directly, we can implement certain strategies to achieve this goal. While some methods require a few lines of code to merge dictionaries, one technique allows us to combine the dictionaries using a single expression. Let's now explore into the different options available to merge two dictionaries in a single expression in Python.
Using Double asterisks (**) Operator
PEP 448, titled "Additional Unpacking Generalizations," introduced a powerful enhancement to the unpacking operator (**), enabling it to be utilized for merging key/value pairs from one dictionary into another. By using dictionary comprehension and the unpacking operator, you can elegantly and efficiently merge two dictionaries in a single expression.
Using the unpacking operator along with dictionary comprehension, you can combine the contents of multiple dictionaries into a new dictionary, making it a concise and expressive approach to handle dictionary merging tasks in Python.
- Python 3.5 or greater
Using Python plus (+) Operator
In Python 2.7, the plus (+) operator can be used to merge two dictionaries into a single expression. Unlike in Python 3.x, where the unpacking operator (**dict) is used for dictionary merging, Python 2.7 offers a straightforward approach using the plus sign.
- Python 2.7
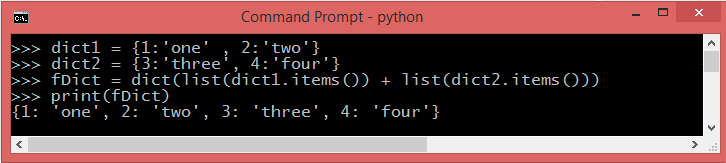
Using ChainMap
ChainMap is a data structure provided by the Python standard library, specifically the collections module, that allows you to view and manage multiple dictionaries as a single entity. It provides a way to combine several dictionaries into a single mapping, enabling you to access and modify the elements from all the dictionaries in a unified manner.
The ChainMap class takes multiple dictionaries as arguments and creates a chain of mappings, where the first dictionary provided takes precedence over the subsequent ones. This means that if a key exists in multiple dictionaries, the value associated with that key in the first dictionary in the chain will be returned.
- Python 3.0 and later
Using Update() method
The update() method in Python dictionaries allows for the merging of elements from another dictionary object or an iterable of key/value pairs into the target dictionary. When using the update() method, if the specified key is absent in the target dictionary, the method adds the element(s) to the dictionary. On the other hand, if the key already exists in the dictionary, the method updates the key with the new value provided in the update operation.
The update() method is a versatile tool that facilitates the modification and expansion of dictionaries, enabling efficient data management and simplifying the handling of key-value pairs in Python programs. By using this method, developers can ensure the integrity and accuracy of dictionary contents, making it a valuable asset in a wide range of programming scenarios.
- Python 2, (or 3.4 or lower)
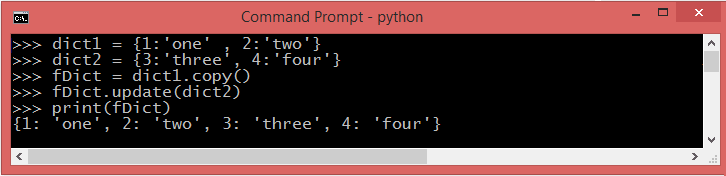
Using | operator
- Python 3.9 and later
As of Python version 3.9, a new dictionary merging and updating feature has been introduced, allowing for a more concise and intuitive syntax. The new features are the union operator for merging dictionaries and the pipe-equal operator = for updating dictionaries.
Using the union operator , you can merge two or more dictionaries into a new dictionary without the need for additional functions or methods. This provides a clear and straightforward way to combine dictionary contents.
Merge multiple dictionaries
If you want to merge more than one dictionary, the simple solution is :
Using comprehension
Comprehensions in Python offer an elegant and succinct approach to create new sequences by utilizing existing sequences that have already been defined. In a pythonic manner, one can utilize comprehensions to efficiently merge two dictionaries. The new syntax introduced in Python 3.9, such as the union operator for dictionary merging and the pipe-equal operator = for dictionary updating, further enhances the expressiveness and readability of the code, enabling developers to accomplish dictionary operations with greater clarity and conciseness. By embracing comprehensions and the latest features, programmers can develop more efficient and maintainable Python code.
Merge Dictionary using Lambda function
A lambda function in Python is capable of accepting any number of arguments, but it can only consist of a single expression. This concise and anonymous function can be effectively employed to merge two dictionaries in Python. By utilizing lambda functions alongside other tools, such as comprehensions and the latest Python features, developers can elegantly and efficiently perform dictionary operations, enhancing the readability and maintainability of their code. Embracing lambda functions allows for the creation of more concise and expressive code, contributing to a more productive and Pythonic coding experience.
Merge n number of Python Dictionaries
The Python itertools module provides a standardized set of iterator building blocks, offering features such as fast and memory-efficient operations. One of the functions in itertools is chain(), which returns a chain object. The chain object behaves like an iterator and seamlessly concatenates multiple iterables together, fetching elements from the first iterable until it is exhausted and then proceeding to the next iterable(s) until all are exhausted. This capability allows for efficient and seamless chaining of iterables, avoiding the need to create large combined data structures and facilitating streamlined and memory-friendly processing of data in Python.
Conclusion
Merging two dictionaries can be accomplished using various techniques. In Python 3.9 and later versions, the union operator can be used for merging dictionaries, while the pipe-equal operator = allows for updating one dictionary with the elements of another. Additionally, the update() method and dictionary comprehension provide alternative ways to merge dictionaries efficiently, providing developers with flexible options to handle dictionary merging tasks effectively.