FTP protocol client in Python
Sending a small file as an email attachment can be suitable for various scenarios, but for larger files, this approach can become cumbersome. FTP streamlines the process of transferring files between different locations, making it more efficient for individuals and businesses. File Transfer Protocol (FTP) is an established open protocol standard widely employed for transmitting and receiving large files. It utilizes the Transmission Control Protocol (TCP) to facilitate the transfer of files from one location to another.
FTP client in Python
Creating an FTP client in Python involves using the built-in ftplib library, which provides functionality to interact with FTP servers. To begin, you need to import the ftplib module and establish a connection to the FTP server using the FTP class. You can then use methods like login(), cwd(), retrbinary(), and storbinary() to perform actions like logging in, navigating directories, uploading, and downloading files. Here's an example of a simple FTP client that connects to a server, lists files, and downloads a file:
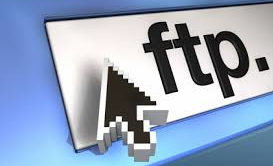
In the above example, replace ftp.example.com, your_username, and your_password with your actual FTP server information. The nlst() method retrieves a list of filenames in the current directory, and retrbinary() is used to download a binary file. This demonstrates the basic process of creating an FTP client using Python's ftplib library.
Conclusion
Creating an FTP client in Python involves utilizing libraries like ftplib to establish connections and transfer files between different locations. By following the appropriate steps, you can create a script that enables efficient file transfer and management over the FTP protocol.