Encryption and Decryption
String Encryption and Decryption
Password storage is a critical aspect of application security, garnering substantial attention in the field. The preferred approach to securely store passwords in modern systems involves generating a hashed representation of the password using contemporary encryption algorithms and robust processes. Hashing employs a one-way function, rendering it irreversible, meaning that once the secure hash algorithm is applied, the original string cannot be retrieved. Unlike storing a hash, the secret key for the symmetric operation is not saved anywhere, minimizing the risk of attackers attempting to recover the passphrase due to its absence. Consequently, the probability of adversaries seeking to find and reconstruct the passphrase is significantly reduced, emphasizing the efficacy of this approach in safeguarding sensitive password information.
RFC2898DeriveBytes Class
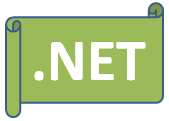
Utilizing RFC2898DeriveBytes with a non-trivial iteration count is a superior approach compared to employing a straight hash function for authentication purposes. The Rfc2898DeriveBytes class serves as a potent tool in generating a derived key, utilizing a base key and other essential parameters. In a password-based key derivation function, the base key is the user's password, while the other parameters consist of a salt value and an iteration count. The incorporation of a non-trivial iteration count enhances the security of the derived key, making it computationally expensive for potential attackers to carry out brute-force attacks or dictionary attacks. This heightened security ensures that password-based authentication is more robust and less susceptible to unauthorized access attempts, further safeguarding sensitive user data from malicious intruders.
PBKDF2
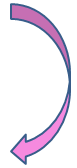
Rfc2898DeriveBytes is an implementation of the PBKDF2 (Password-Based Key Derivation Function 2) algorithm. PBKDF2 employs a pseudorandom function and allows for a configurable number of iterations to derive a cryptographic key from a given password. The key derivation process is designed to be challenging to reverse while also being configurable to be computationally slow, making it highly suitable for password hashing scenarios. The openness of PBKDF2's details ensures transparency and allows security experts to analyze and scrutinize its effectiveness. The primary objective of PBKDF2 is "key stretching," which significantly increases the complexity of generating or reversing the hash, thereby enhancing the security of the entire process. The .NET Framework conveniently abstracts the intricacies of the algorithm, sparing developers from the burden of implementation details and facilitating their focus on secure password hashing practices.
AES
AES (Advanced Encryption Standard) does not have specific vulnerabilities related to related key attacks. In fact, AES is widely regarded as a secure and robust encryption algorithm and is widely used in various applications and systems for ensuring data confidentiality.
AES is a symmetric encryption algorithm, meaning that the same key is used for both encryption and decryption. It has undergone extensive cryptographic analysis and has withstood scrutiny from the security community over the years.
AES is designed to resist known cryptographic attacks, including related key attacks, differential cryptanalysis, and linear cryptanalysis. Related key attacks occur when an attacker can exploit a specific relationship between multiple keys and encrypted data. However, AES is specifically designed to thwart such attacks.
To ensure the security of AES encryption, it is essential to use a strong and unique key for each encryption operation, and the key should be properly managed and protected to prevent unauthorized access.
As with any cryptographic system, the security of AES also depends on the strength of the encryption key and the implementation of the algorithm. Inadequate key management practices or implementation flaws could potentially weaken the overall security of the system.
System.Security.Cryptography
The System.Security.Cryptography namespace provides cryptographic services, including secure encoding and decoding of data
Encrypt and Decrypt a String
From the following program you can learn how to Encrypt a string and Decrypt an Encrypted String
Source Code | C#
Password Security and Encryption
Source Code | Vb.Net
Limitations
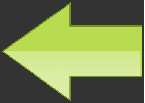
The built-in .NET implementation of Rfc2898DeriveBytes restrict the user to one pseudorandom function - HMAC with SHA-1. This is acceptable in most cases today, but in the future, a more complex hashing function may be required. Moreover, the .NET Compact Framework does not support Rfc2898DeriveBytes.
Conclusion
It's important to keep AES and its implementation up-to-date with the latest security standards and best practices to maintain its resilience against potential attacks. Regular security audits and reviews of cryptographic systems are essential to identify and address any potential vulnerabilities.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#