Enum with Switch.Case
Enumeration and Switch Case
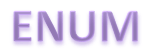
Numerical constants, commonly known as "nums," exemplify the tenet of strong typing in the field of programming. Their resolute adherence to specific data types furnishes utmost precision and reliability to programming endeavors. When confronting scenarios that demand steadfast and unvarying values, these constants prove exceedingly valuable. Their unwavering nature ensures that once assigned, their values remain impervious to alteration throughout program execution, bestowing stability upon the codebase.
In situations necessitating the use of numerous constant integral values, nums emerge as an efficient and elegant solution, offering the facility to consolidate these values into a singular variable with unparalleled ease and clarity. This fusion not only streamlines the code but also enhances its readability, making it more comprehensible to developers and collaborators alike. The judicious implementation of nums exhibits the virtuosity of skilled programmers, as it underscores their commitment to producing efficient, maintainable, and dependable software solutions.
Syntax
Switch Case
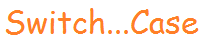
In C#, you can use switch...case statements with enum values to create more concise and readable code when handling different cases based on enum values. To demonstrate this, let's declare an enum called BackColors in a C# program:
In this program, we define an enum BackColors with five color values: Red, Green, Blue, Yellow, and White. Then, we use a switch...case statement to handle different cases based on the value of the selectedColor variable, which is of type BackColors.
When you run the program, it will output:
This is because we have assigned BackColors.Green to the selectedColor variable, and the corresponding case BackColors.Green is matched in the switch...case statement, resulting in the message "Selected color is Green." You can change the value of selectedColor to any other BackColors value, and the switch...case statement will handle the corresponding case accordingly.
Conclusion
Using switch...case with enums allows you to create more concise and maintainable code when dealing with multiple cases based on enum values, making your code easier to understand and modify in the future.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#