Java Enum (Enumerations)
An enum in Java is indeed similar to any other class but with a distinct purpose: defining a predefined set of instances or members. It serves as a data type that allows for a more readable and reliable representation of each member within a specific type or category.
Enums are particularly useful when there is a need to define a finite set of values that a variable can take. For instance, in the temperature levels, an enum can be created to represent the possible values of High, Medium, and Low. By using an enum, the code becomes more expressive and self-explanatory, as these meaningful names replace arbitrary numerical or textual representations.
Enums can have methods, constructors, and properties just like regular classes, making them highly flexible. Each enum instance is treated as a separate object, enabling the inclusion of additional behavior or attributes specific to each member. This makes enums a powerful tool for modeling and representing predefined sets of related values in a structured and intuitive manner.
The main advantage of Enum is that it make your code more explicit, less error-prone and in a self-documenting way.
When should I use?
Enum types prove to be a valuable asset whenever the requirement arises to portray a definitive and unchanging collection of constants. Enum types find their purpose when a variable, particularly a method parameter, is restricted to a limited set of possible values. For instance, scenarios such as representing the days of the week (Sunday, Monday, etc.) or cardinal directions (NORTH, SOUTH, EAST, and WEST) call for the utilization of enums.
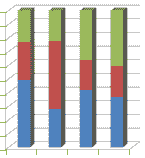
By utilizing an enum instead of a class, developers can effectively handle situations where the number of instances remains fixed and enumerable. Enum types offer a concise and straightforward solution in such cases, eliminating the need for a full-fledged class structure. This streamlined approach enhances code readability and maintains a clear and intuitive representation of the predefined set of instances.
The versatility of enum types allows for seamless integration within various components of a Java program. Whether it is using enum constants as method parameters or incorporating them as variables, enums provide a robust mechanism to enforce type safety and reduce the likelihood of errors resulting from incompatible or incorrect values.
Enum switch...case Example :
An enum type can also be employed within a switch...case statement, similar to how one would use primitive data types like int or char. This feature allows for a more expressive and structured approach when dealing with a set of predefined values.
By utilizing an enum within a switch...case statement, developers can easily handle different cases based on the specific enum constant. Each enum constant can be associated with a distinct block of code, enabling efficient branching and decision-making within the program flow.
This usage of enums within switch...case statements brings multiple benefits. It enhances code readability by providing a clear and concise representation of the different cases that can be handled. Additionally, it helps enforce type safety, as the switch statement is constrained to the specific enum type and its valid constants. This eliminates the possibility of accidental mismatched or unsupported values.
The following program shows how to use Enum in switch...case statement.
Enum if...esle Example :
You can also use an enum type in a Java if..else statement. The following program shows how to use Enum in if..else statement.
Enum for loop Example :
The following Java program shows how to use Enum in for loop.
Points to remember for Java Enum
- Enum considered more type-safe than constants: One of the common use of enums is to prevent the possibility of an invalid parameter.
For example, consider a normal method like the following:
The above method is not only ambiguous (do day indexes start at 1, or at 0?), but you can give down-right invalid data like greater than 7 or negative numbers. Instead, if you have an enum DAYS with Sunday, Monday, etc. the signature becomes:
Code calling this method will be far more readable, and can't provide invalid data.
Utilizing enums instead of constants offers significant advantages in terms of type safety. When a function expects an enum as a parameter, the compiler enforces strict adherence to the expected data type. If anything other than an enum is passed, the compiler generates an error, highlighting the inconsistency and preventing potential runtime issues.
In contrast, when constants are used, a wide range of data is accepted, including values that may be invalid or unintended for the given context. This opens the door to potential bugs or unexpected behavior during program execution.
By employing enums, developers establish a well-defined and restricted set of permissible values. This clarity in data representation ensures that only valid and relevant values can be passed to functions or assigned to variables. The compiler's ability to verify the data type at compile-time enhances the robustness and reliability of the codebase.
- java.util.EnumSet & java.util.EnumMap: Two classes have been added to java.util to support enum: EnumSet and EnumMap. They are high performance implementation of the Set and Map interfaces respectively.
- All constants defined in an enum are public static final. Since they are static, they can be accessed via EnumName.instanceName.
- An enum can be declared outside or inside a class, but NOT in a method.
- Adding new constants on Enum in Java is easy and you can add new constants without breaking the existing code.
Conclusion
Java Enum (enumerations) is a special data type that allows you to define a collection of constants with a fixed set of values. Enums in Java are used to represent a group of related constants, making the code more readable, type-safe, and maintainable. Enums provide a better alternative to using integer constants or string constants in situations where a variable can only take a limited number of predefined values.