Java :public static void main(String[] args)
The main() method holds significant importance in Java programming as it acts as the entry point for the program's execution, allowing it to be run from the command line or any Java runtime environment. While compiling a Java program, the presence of a main() method is not strictly required, but during execution, the JVM searches for this method and initiates program execution from there.
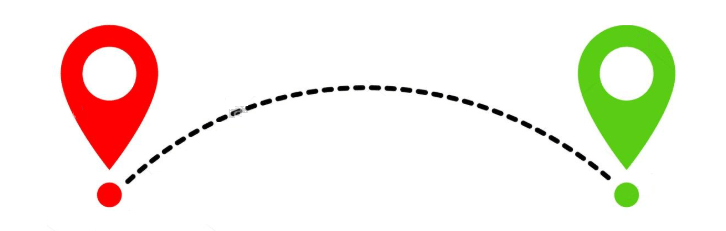
The main() method in Java must have a public access modifier, enabling it to be accessed from outside the class. Being a static method, it can be called without creating an instance of the class. Additionally, it does not return any value and accepts a String array as a parameter, allowing command-line arguments to be passed to the program.
- The modifiers public and static can be written in either order (static public or public static), but the convention is to use public static as shown above.
- You can define a main() method with any access modifier or with/without static keyword, but then it is not a valid main() method, as the main method which the JVM uses as an entry-point should be defined as such.
- You can name the argument anything you want, but most developers choose "args" or "argv".
You can write a program without defining a main() it gets compiled without compilation errors . But when you execute it, a run time error is generated saying "Main method not found".
Let's split it and understand one by one.
public
The main() method in Java has a public access specifier, making it globally accessible. This is crucial because the Java Runtime Environment (JRE) calls this method from outside of your current class. It's essential to keep the main() method public; otherwise, access restrictions will prevent it from being executed by any program.
static
The main() method in Java must be declared as static. By making the main() method static, it can be invoked directly by the JVM without the need to create an instance of the class. This is essential because the JVM needs a starting point to execute the program, and it does so by calling the main() method without instantiating the class.
If the main() method were not static and the JVM had to instantiate the class, there could be ambiguity if the class had multiple constructors, especially if they took different arguments. Making the main() method static eliminates this ambiguity and ensures that the JVM can directly call the method to start the program execution.
void
The main() method in Java is declared with a return type of "void" to indicate that it does not return any value. The purpose of the main() method is to serve as the entry point for the Java program, and it is invoked by the Java Virtual Machine (JVM) to start the execution of the program.
When the main() method terminates, the Java program also terminates, regardless of whether it has spawned new threads. Any return value from the main() method would not have any impact on the program's execution or termination because the JVM does not process the return value of the main() method.
If you attempt to add a return statement with a value in the main() method, the Java compiler will raise a compilation error because the main() method must have a return type of "void" and cannot return any value.
![Understanding public static void main(String[] args) in Java](img/main-method.png)
main()
It's just the name of method or a function name. This name is fixed and as it's called by the JVM as entry point for an application. It's not a keyword.
String args[]
The Java main() method accepts arguments of type String when you run your Java application. These arguments are passed to the main() method as a string array. The string array stores a collection of strings, separated by spaces, which can be provided as input to the program when executed on the terminal.
In the main() method, the brackets [] can be placed after the type or the variable to indicate that it is an array of strings. However, it is generally preferred to place the brackets after the type to indicate that the main() method accepts an array of strings as its argument.
If you wanted to output the contents of args , you can just loop through them like this...
Run the java program with arguments like the following:
Conclusion
The Java main() method is a special entry point for running Java programs. It must be declared as "public static void main(String[] args)" and serves as the starting point for the Java program's execution, accepting command-line arguments as a string array.