How to Set Path and Classpath in Java
Java PATH and Java CLASSPATH are environment variables that often confuse beginners in Java programming. These variables are set at the operating system level and play crucial roles in how Java applications are executed and how they find and load external resources. Understanding the differences between these two variables is essential for effectively managing Java development environments and ensuring smooth execution of Java programs. Properly configuring and utilizing these environment variables will enhance the efficiency and reliability of your Java projects.
What are Environment Variables ?
In various operating systems, environment variables serve as a means of conveying configuration details to applications, including those written in Java. These variables are represented as key/value pairs, with both the key and value being strings. However, the implementation and behavior of environment variables can differ subtly across different operating systems. For instance, Windows treats variables in a case-insensitive manner, whereas UNIX systems are case-sensitive.
Additionally, the conventions for naming environment variables also vary, with common practice involving uppercase letters and underscores to separate words, as exemplified by "JAVA_HOME". Understanding these nuances is vital for effectively managing and utilizing environment variables in Java applications across different platforms.
Environment Variables examplesThe proper configuration of environment variables, particularly PATH, CLASSPATH, and JAVA_HOME, is critical for smooth installation and execution of Java applications. Errors in setting these variables can lead to various issues and hinder the functionality of Java programs. The PATH variable specifies directories in which the operating system should search for executable files, including Java executables. CLASSPATH defines the locations where Java should search for user-defined classes and resources. Lastly, JAVA_HOME points to the root directory of the Java Development Kit (JDK). Accurate settings of these environment variables ensure that Java applications can be located, executed, and function as intended, eliminating potential problems and ensuring a hassle-free experience with Java.
How to set Java path in Windows
The Java PATH environment variable plays a crucial role in specifying the locations of executable binaries. When running a Java program from the command line, the operating system searches for the required binaries in the directories listed in the PATH variable. To execute Java programs, the 'java.exe' binary is used, while for compiling Java code, 'javac.exe' is utilized. These executable files are typically found in the 'bin' folder of the Java installation directory.
By setting the PATH variable appropriately, you ensure that the operating system can locate and execute these binaries without any issues. The PATH variable can be set temporarily for a specific command prompt session or permanently to be available for all future sessions. Properly configuring the Java PATH environment variable is essential for seamless execution of Java programs from the command line.
Set path from Windows command line (CMD)
Open command prompt and type the following on command prompt.
Example

Set Permanent Path of Java in Windows
In Windows inorder to set
- Step 1: Right Click on MyComputer and click on properties
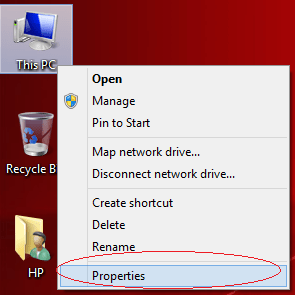
- Step 2: Click on Advanced System Setting
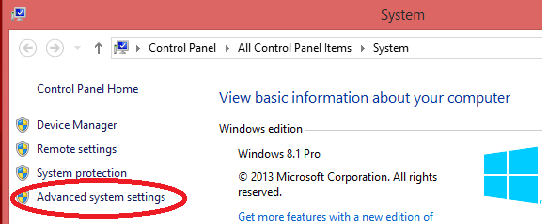
- Step 3: Select Advanced Tab and Click on Environment Variables
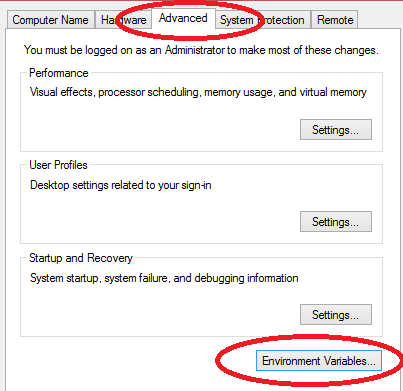
- Step 4: Then you get Environment Variable window and Click on New...
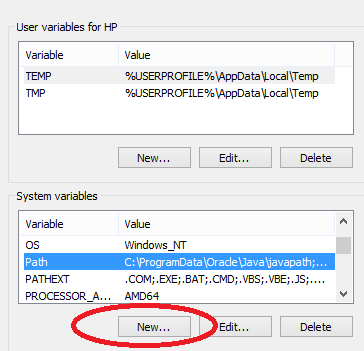
Then you get a small window "New System Variable" and there you can set "Variable Name" and "Variable Value". Set Variable Name as "path" and Variable Value as "your jdk path".
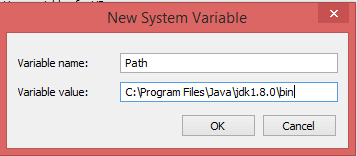
Click "OK" button. Now you set your Java Path and next is setting up ClassPath.
How to Set Classpath for Java on Windows
The Java CLASSPATH environment variable is crucial for specifying the location of user-defined classes and packages that are needed by Java applications. It is a parameter used by the Java Virtual Machine (JVM) and the Java compiler to locate the compiled Java class files (.class files). The CLASSPATH can be set either through the command-line or as an environment variable.
By default, if the CLASSPATH is not set, it is assumed to be the current directory. However, it is essential to explicitly include the current working directory (represented by a period '.') in the CLASSPATH to ensure that the JVM searches for classes in the current directory.
In Windows inorder to set ClassPath :
Repeat the above steps: Steps1 to Step4 .
Then you get a small window "New System Variable" and there you can set "Variable Name" and "Variable Value". Set Variable Name as "ClassPath" and Variable Value as "your class path" (ex: C:\Program Files\Java\jdk1.8.0\lib\* ).
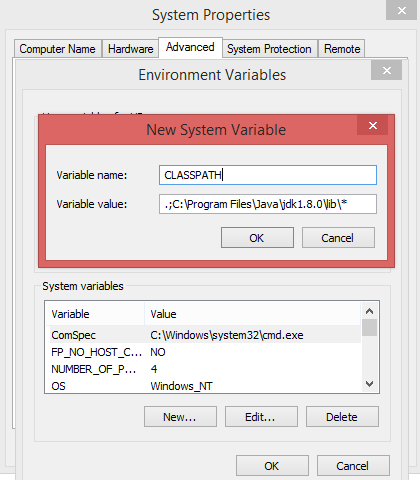
Using wildcards in java classpath
Wildcards were introduced in Java 6 to enhance classpath configurations. Class path entries can now include the basename wildcard character "", which effectively represents a list of all the files in the directory with the extensions .jar or .JAR. For instance, a classpath entry containing just the "" wildcard will expand to encompass all the jar files in the current directory, simplifying the process of specifying multiple jar files in the classpath. This feature provides a more convenient and concise method to include multiple files in the classpath without having to enumerate each file individually.
Example
where %MAINCLASS% is the class containing your main method.
How to use a wildcard in the classpath to add multiple jars
where %MAINJAR% is the jar file to launch via its internal manifest.
If you need only specific jars, you will need to add them individually. The classpath string does not accept generic wildcards like Jar*, *.jar, hiber* etc.
Example
The following entry does not work:
Correct entry is :
Conclusion
Properly configuring the CLASSPATH is vital for Java applications to access user-defined classes and packages and to ensure smooth execution of Java programs that rely on external classes and libraries. Incorrect settings of the CLASSPATH can lead to errors such as "class not found" during the execution of Java applications.