How to send email using Python
Sending email using Python involves utilizing the built-in smtplib library for handling the email sending process and the email library for constructing the email content. Below is a step-by-step explanation along with an example:
Import Libraries
Begin by importing the required libraries: smtplib for sending emails and email for constructing the email content.
Set Up the Email Content
Create an instance of MIMEMultipart to build the email structure. Set the From, To, Subject, and the email body using MIMEText.
Connect to SMTP Server
Establish a connection to the SMTP (Simple Mail Transfer Protocol) server of your email provider. For example, if you are using Gmail, you would connect to Gmail's SMTP server.
Login to Your Email Account
Login to your email account using your email address and password. Make sure to use an "App Password" if you have two-factor authentication enabled for your email account.
Send the Email
Send the constructed email using the sendmail method. The email should be converted to a string using .as_string().
Close the Connection
After sending the email, close the connection to the SMTP server.
Sending Emails With Python | Python
Remember to replace placeholders like your_email@gmail.com, recipient@example.com, and your_email_password with your actual email address, recipient's email address, and email password, respectively.
SMTPAuthenticationError when sending mail using gmailSometimes when you send email using gmail address you can see some error message like this:
It is because Google blocks sign-in attempts from apps which do not use modern security standards (mentioned on their support page). You can however, turn on/off this safety feature by going to the link below:
Go to this link and select Turn On
https://www.google.com/settings/security/lesssecureappsMoreover google block an ip when you try to send a email since a unusual location, so, you can unblock in the next link
https://support.google.com/accounts/answer/6009563and clicked in
accounts.google.com/DisplayUnlockCaptcha .
Gmail SMTP Settings
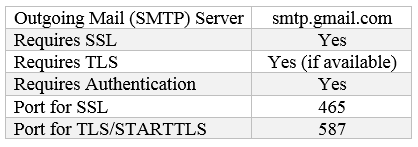
If you use Gmail with your work or school account, check with your administrator for the correct SMTP configuration.
Conclusion
Sending emails using Python involves using the smtplib library for sending and the email library for constructing the email content. Import the necessary modules, set up the email structure with sender, recipient, subject, and body, establish a connection to the SMTP server, login to your email account, send the email, and close the connection. It's a straightforward process that requires setting up the email content, connecting to the server, and using the sendmail method to send the constructed email.