Pyhon Socket programming
Socket programming is a fundamental component of computer network programming, enabling bidirectional communication between programs on a network. Python simplifies socket programming through its socket module, granting access to the widely supported BSD socket interface on various operating systems, including Unix, Windows, Mac OS X, BeOS, OS/2, and potentially others.
The Python Socket Programming has two sections:
- Python Server Socket Program
- Python Client Socket Program
Python Server Socket Program
The Server Socket Program is a console-based Python application that functions as a server, actively listening for client requests on Port No. 8080.
Once the Server Socket accepts a request from the Client side, it reads the data sent by the client and also writes a response back to the connected client program.
Python Client Socket Program
The client socket is connected to port 8080 of the Python server socket program, as well as the IP address ("127.0.0.1") of the server machine. We utilize the IP address 127.0.0.1 in this context because the server and client are running on the same machine. However, if the client program is running on a different machine, you should provide the IP address of that specific machine.
When the Python client program starts, it establishes a connection with the Python server socket program and waits for input from the client side. Once a message is typed, it is sent to the server, and subsequently, the client can receive and view the reply messages from the server side as well.
Python Socket Client Example(client.py)How to run this program ?
To implement Python Server Socket Program (Server.py) and Python Client Socket Program (client.py) in separate files, follow these steps:
- Complete the coding for both programs.
- Launch the Python Server Socket Program from the command prompt (console) once coding is finished.
- You will receive informative messages such as "Server Started..." and "Waiting for client request..." on the console where the server program is running.
To proceed, initiate the Python Client Socket Program from the command prompt (console) on either the same computer or other computers within the same network. Upon starting the client program, it will establish a connection to the server and transmit the message "This is from the client" from the client side. After the server receives the message, it will respond to the client with the message "Successfully Connected to Server!!".
At this point, you will observe the communication between the client program and the server program, demonstrating their interaction and exchange of information.
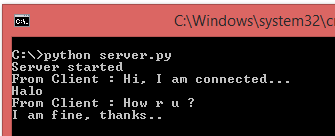
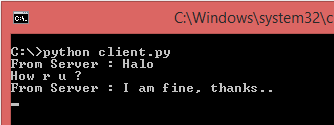
Please note that if you are running the client program on a different computer within the same network, you need to replace "127.0.0.1" in the client program with the IP address of the server machine.
Asynchronous Socket Programming (Read-Write both side)
In the above example, you can just send one message from client as well as just one return message from server also. If you want to connect and communicate client and server repeatedly, you should implement a while loop for sending and receiving data each other.
Python Server Socket Example (Server.py)How to run this program ?
To proceed, follow these steps:
- Create two separate files: "server.py" for the Python Server Socket Program and "client.py" for the Python Client Socket Program.
- Once you have finished coding, initiate the Python Server Socket Program by starting it from the command prompt or console.
- Upon successful execution, you will receive the messages "Server Started..." and "Waiting for client request..." displayed in the console where the server program is running.
The next step is to start the Python Client Socket Program from the command prompt or console, either on the same computer or on other computers within the same network. When you start the client program, it will establish a connection to the server and send the message "This is from the client" from the client side. After the server receives the message from the client side, it will wait for input from the server side.
At this point, you can enter a message from the server side and press the Enter key. Simultaneously, the client will receive the message and wait for input from the client side. Once again, you can enter a message from the client side and press the Enter key. This back-and-forth communication between the server and client can continue repeatedly.
You can continue this communication until you send the message "bye" from the client side, indicating the end of the communication.
Conclusion
Python socket programming enables communication between networked devices by providing a mechanism for creating client and server applications that can exchange data over TCP/IP connections.