Web Scraping in Python
Reading selected webpage content using Python involves utilizing the requests library, which allows you to make HTTP requests to web servers and retrieve web content. Here's a step-by-step explanation with an example:
Install the Requests Library
If you haven't already, you need to install the requests library. You can do this using the following command in your terminal or command prompt:
Import the Library
Import the requests library at the beginning of your Python script:
Send HTTP Request
Use the get() method from the requests library to send an HTTP GET request to the desired webpage. Provide the URL of the webpage you want to retrieve as an argument.
Check Response Status
You can check the status code of the response to ensure the request was successful. A status code of 200 indicates a successful request.
Extract Selected Content
You can use the response.text attribute to access the raw HTML content of the webpage. You can then use techniques like string manipulation, regular expressions, or parsing libraries like BeautifulSoup to extract the specific content you're interested in.
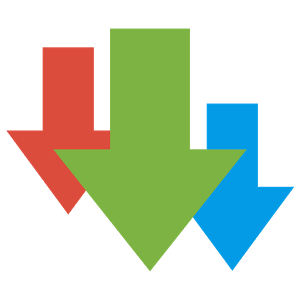
Web Scraping | Python
Here's a simplified example of how you might retrieve and print the title of a webpage using the requests library and BeautifulSoup for parsing:
This example demonstrates the basic process of retrieving webpage content using Python. Depending on your specific needs, you can further enhance the parsing and content extraction techniques to gather the information you require from the webpage.
Conclusion
Reading selected webpage content using Python involves using the requests library to send an HTTP GET request to a webpage, retrieving its HTML content. The response.text attribute provides access to the raw HTML, which can be processed further using parsing techniques like BeautifulSoup to extract specific information such as titles, text, or data from the webpage. This process allows developers to programmatically retrieve and manipulate webpage content for various purposes.