Detecting arrow keys
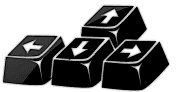
Most Windows Forms applications primarily handle keyboard input through the diligent management of keyboard events. By adeptly utilizing the KeyDown or KeyUp events, one can effectively detect the majority of physical key presses. The sequence of key events unfolds in a carefully organized manner, ensuring smooth and seamless processing. Key events occur in the following order:
- KeyDown
- KeyPress
- KeyUp
Handle arrow key events in c# and vb.net
Arrow Key movement on Forms c# and vb.net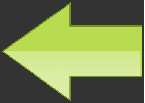
In the majority of scenarios, the standard KeyUp, KeyDown, and KeyPress events serve as sufficient means to capture and manage keystrokes adeptly. Nevertheless, there are instances where specific controls may not trigger these events uniformly for all keystrokes and circumstances. Notably, the KeyPress event is raised solely for character keys when they are both pressed and subsequently released. Conversely, noncharacter keys do not prompt the KeyPress event to be raised, in contrast to KeyDown and KeyUp events, which are duly raised for both character and noncharacter keys. Hence, it is vital to be cognizant of these distinctions while handling keystrokes in different contexts.
Handle arrow key events in Windows Forms in C# and VB.Net
To efficiently capture keystrokes within a Forms control, a recommended approach involves creating a new class derived from the base class of the desired control. In this subclass, you override the ProcessCmdKey() method, which allows you to intercept and handle command keys before they are processed by the control. This technique provides greater flexibility in customizing the control's behavior with respect to keyboard input.
Syntax:
C#:
VB.Net:
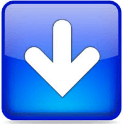
The ProcessCmdKey method is indeed called during message preprocessing to handle command keys, and it is specifically invoked when the control is hosted in a Windows Forms application or as an ActiveX control. This method provides a way to intercept command keys before they are dispatched to the control's standard handling routines.
How to detect which Arrow Key is pressed ?
C# code for capture arrow keys.It's important to understand that message preprocessing occurs at a lower level than standard event handling. When a control receives a message, the message is first preprocessed, and if a command key (e.g., shortcut key) is detected, the ProcessCmdKey method is called to allow the control to handle the key before raising higher-level events like KeyDown, KeyPress, and KeyUp.
It's worth noting that the ProcessCmdKey method is specific to Windows Forms controls and is not available for all controls in other user interface frameworks. Additionally, if you are developing custom controls for Windows Forms or ActiveX applications, you can utilize this method to provide custom keyboard handling tailored to your control's needs.
Here's a brief summary of the key points about ProcessCmdKey:
- ProcessCmdKey is called during message preprocessing to handle command keys (e.g., shortcuts) before standard event handling.
- It is available for controls in Windows Forms and ActiveX applications.
- By overriding this method, you can intercept and handle specific command keys according to your control's requirements.
- It provides a way to implement custom keyboard handling for your controls.
Conclusion
Always consider the specific context and use case when employing the ProcessCmdKey method, and be aware that it is most effective for customizing keyboard behavior within Windows Forms applications or when hosting controls as ActiveX components.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#