Autocomplete ComboBox
A ComboBox is a graphical user interface element that seamlessly integrates a text box with a ListBox, offering users the dual functionality of selecting pre-existing items from the list or entering new values. Notably, since the release of Visual Studio 2005, certain controls have been enriched with the Autocomplete feature, and this includes the ComboBox controls. The Autocomplete feature significantly enhances the user experience by providing predictive suggestions as the user types in the ComboBox, saving time and reducing errors during data entry or selection.
Autocomplete ComboBox
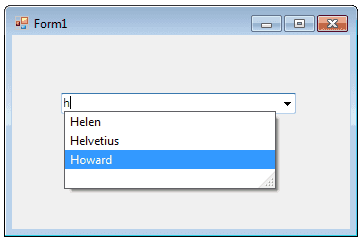
The AutoComplete properties, namely AutoCompleteCustomSource, AutoCompleteMode, and AutoCompleteSource, enable the functionality of a TextBox that automatically completes user entry strings by intelligently comparing the initial letters being entered to the prefixes of all strings in a designated data source. While the utilization of the AutoCompleteCustomSource property is discretionary, it is essential to set the AutoCompleteSource property to CustomSource to utilize the power of AutoCompleteCustomSource as a data source, which can encompass databases, lists, or other relevant sources.
In simpler terms, these AutoComplete properties facilitate the implementation of a TextBox with an automatic suggestion feature that predicts and completes user input based on the initial characters entered. If desired, developers can provide a custom set of suggestions using the AutoCompleteCustomSource property, but it is crucial to indicate CustomSource in the AutoCompleteSource property to enable the use of this custom data source effectively.
C# Autocomplete ComboBox
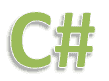
The following program displays a C# Autocomplete ComboBox that select the values from Database and add to AutoCompleteStringCollection of the Autocomplete ComboBox.
Source Code | C#
VB.Net Autocomplete ComboBox
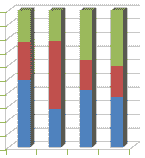
The following program displays a VB.Net Autocomplete ComboBox that select the values from Database and add to AutoCompleteStringCollection of the Autocomplete ComboBox.
Source Code | VB.Net
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#