Autocomplete TextBox
Since the release of Visual Studio 2005, an array of controls has been integrated with the highly efficient Autocomplete feature, with TextBox controls being one of the beneficiaries. This Autocomplete functionality empowers developers with three significant properties: AutoCompleteCustomSource, AutoCompleteMode, and AutoCompleteSource. These properties collectively enable the implementation of a TextBox that effortlessly predicts and completes user input strings by intelligently comparing the entered prefix letters to the prefixes found within a designated data source.
With the AutoCompleteCustomSource property, developers gain the ability to define a custom set of options that the Autocomplete feature utilizes to offer predictive suggestions to users as they type. This empowers developers to tailor the Autocomplete functionality to suit specific application requirements, ensuring a more personalized and seamless user experience.
Autocomplete TextBox
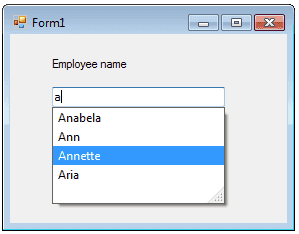
The AutoCompleteMode property enables developers to precisely control the behavior of the Autocomplete feature. By selecting the appropriate mode, developers can dictate when and how the Autocomplete suggestions appear, optimizing the feature's responsiveness and adaptability to different user interaction patterns.
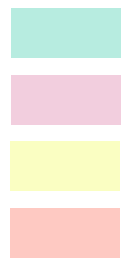
The AutoCompleteCustomSource property proves to be particularly beneficial when working with TextBox controls that frequently involve the input of specific string sources, such as URLs, addresses, and other frequently used data. By utilizing this property, developers can establish a custom list of values, tailored to the unique needs of the application. This allows the Autocomplete feature to present relevant suggestions based on the user's input, streamlining the process of data entry and ensuring accuracy by offering pre-defined options that match the specific context.
On the other hand, the AutoCompleteMode property plays a vital role in determining how the auto-complete candidates are displayed to the user. This property empowers developers to choose from a variety of display modes, enabling them to control when and how the Autocomplete suggestions appear to the user as they type in the TextBox control. By selecting an appropriate mode, developers can fine-tune the user experience to best suit the application's requirements and the preferences of its users.
C# Autocomplete TextBox
The following C# program add some data values to AutoCompleteStringCollection and perform as an Autocomplete TextBox
The following C# program connect to database and add Dataset values to AutoCompleteStringCollection and perform as an Autocomplete TextBox.
VB.Net Autocomplete TextBox

The following VB.Net program add some string values to AutoCompleteStringCollection and display as Autocomplete TextBox while entering text.
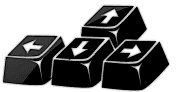
The following VB.Net program connect to database and add Dataset values to AutoCompleteStringCollection and display as an Autocomplete TextBox while entering values to TextBox control.
Conclusion
The AutoCompleteCustomSource property enables the setup of a custom list of values, which is particularly useful when dealing with frequently entered string sources. Meanwhile, the AutoCompleteMode property provides the flexibility to customize how the Autocomplete suggestions are presented, enhancing the user's interaction with the application and making the process of data entry more efficient and user-friendly.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#