String Vs StringBuilder | C#
In C#, both String and StringBuilder are used to work with text and represent sequences of characters. However, they have different characteristics and are optimized for different scenarios. Understanding the differences between String and StringBuilder is important to choose the appropriate one for your specific use case.
String
String is an immutable data type, which means that once a string object is created, its value cannot be changed. Whenever you perform operations on a string (e.g., concatenation, substitution), a new string object is created in memory, and the original string remains unchanged. This can lead to performance and memory issues when dealing with a large number of string manipulations.
In this example, the fullName variable contains the concatenated string "Smith Warner," but two intermediate string objects are created in memory before the final result.
StringBuilder
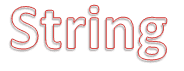
StringBuilder, on the other hand, is a mutable data type specifically designed for efficient string manipulation. It allows you to modify the contents of a string without creating new objects each time, making it more efficient when dealing with extensive string concatenation or modification operations.
In this example, the StringBuilder object sb is used to build the fullName string. The Append method is used to concatenate the individual parts, and ToString() is used to get the final result as a string. Only one string object is created in this process.
When to use String or StringBuilder
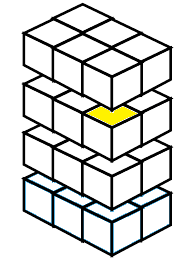
Use String when you are dealing with a fixed text that won't change during the lifetime of the program, or when you need to perform limited string manipulations. Since strings are immutable, they are safe to share between multiple threads.
Use StringBuilder when you are dealing with extensive string manipulations, such as concatenating multiple strings in a loop, building dynamic SQL queries, or modifying large text files. StringBuilder is more memory-efficient and performs better in these scenarios, as it minimizes the overhead of creating new string objects.
In this loop example, using StringBuilder reduces memory usage and improves performance compared to repeatedly concatenating strings with the + operator or using string.Format().
Conclusion
If you have a small number of string manipulations or deal with fixed text, using String is appropriate. However, for extensive string manipulation or frequent concatenations, StringBuilder is the better choice to avoid unnecessary memory and performance overhead.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#