.Net Frequently Asked Questions (C#, Asp.Net and VB.Net)
The subsequent sections encompass a compilation of Frequently Asked Questions (FAQs) comprising the latest and most authentic interview questions related to Microsoft .NET Framework and its associated technologies, including Asp.Net, C#, and VB.Net. These carefully curated questions serve as valuable resources for gaining in-depth knowledge and insights into the intricate aspects of these technologies, making them indispensable for professionals seeking to enhance their proficiency in the Microsoft .NET ecosystem
What is the use of using statement in C# ?
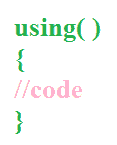
The using statement is primarily used for automatic resource management. It provides a convenient and efficient way to work with objects that implement the IDisposable interface. The primary purpose of the using statement is to ensure that any resources acquired by an object that implements IDisposable are properly released or disposed of, regardless of whether an exception occurs during the code execution.
How the using statement works in C#?
Automatic Resource Management
When you create a variable using the using statement, the compiler generates a try-finally block under the hood. The resource is acquired within the using block and is automatically released or disposed of at the end of the using block, ensuring proper resource management.
IDisposable Interface
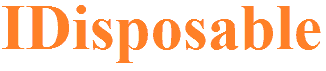
The IDisposable interface defines a method named Dispose(), which is responsible for releasing any unmanaged resources held by the object. This could include file handles, network connections, or any other resources that are not automatically managed by the .NET runtime.
Here's the basic syntax of the using statement in C#:
Suppose we want to read the contents of a file using the FileStream class. The FileStream class implements IDisposable, so we can use the using statement to ensure that the file handle is properly closed when we are done with it:
When working with a database connection, we can also use the using statement to ensure that the connection is properly closed and returned to the connection pool:
Conclusion
In both examples, the using statement ensures that the resources (FileStream and SqlConnection) are properly disposed of, even if an exception occurs during the execution of the code within the using block. This helps in preventing resource leaks and improves the overall robustness and reliability of the application.
- String and System.string
- Dictionary in C#
- String to byte Array
- Detecting arrow keys
- Enum with Switch.Case
- Passing Data
- Autocomplete TextBox
- Autocomplete ComboBox
- Convert enum to List
- MemoryStream to file
- Parse XML - XmlReader
- Parse XML - XmlTextReader
- Parse XML - XmlDocument
- File.Exists Method
- Decimal vs Double vs Float
- String to DateTime
- ComboBox text and value
- sort array
- Image to Byte Array
- Numeric Only TextBox Control
- NullReferenceException in C#
- Custom Exception in C#
- Throwing Exceptions - C#
- String Vs StringBuilder | C#
- ByteArray from Stream c#
- Remove spaces from string | C#
- Remove new line from a string in C#
- Remove non alphanumeric | C#
- Character Encoding
- Connect C# to MySQL
- Byte array to string in C#
- What is an IP Address ?
- Running a .BAT file
- Rounding to 2 Decimal Places
- Multiple Lines String
- Encryption and Decryption
- Datatype of a variable
- Aautoscroll multiline TextBox
- Difference between forEach and for loop
- Convert byte array to hex string
- Catch multiple exceptions at once: C#