Dictionary in C#
In C#, a Dictionary<TKey, TValue> is a collection that stores key-value pairs, where each key is unique. When iterating over a dictionary, there are several methods available, each with its advantages and use cases. Here are the best ways to iterate over a dictionary in C#:
Using foreach loop with KeyValuePair<TKey, TValue>
The most common and straightforward way to iterate over a dictionary is by using a foreach loop. The loop iterates through each key-value pair in the dictionary, represented by the KeyValuePair<TKey, TValue> structure.
Using foreach loop with var
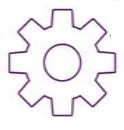
In C# 3.0 and later versions, you can use the var keyword to infer the type of the loop variable automatically. This approach simplifies the code and makes it more concise.
Using foreach loop on keys or values
If you only need to iterate over the keys or values of the dictionary, you can use the Keys or Values properties of the dictionary.
Using LINQ methods (e.g., foreach, Select, Where, etc.)
The LINQ (Language-Integrated Query) library provides a set of extension methods that can be used to query and manipulate collections, including dictionaries. You can use LINQ methods like Where, Select, and ForEach to filter or project dictionary elements as needed.
Conclusion
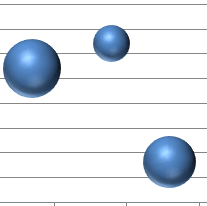
Choosing the best method for iterating over a dictionary depends on your specific use case. If you need to work with both keys and values, using the foreach loop with KeyValuePair<TKey, TValue> is generally the most suitable and straightforward approach. However, if you only need to process keys or values, you can use the Keys or Values properties. Additionally, if you require more complex filtering or projection, the LINQ methods provide powerful and flexible options for working with dictionaries.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#