Image to Byte Array
In numerous situations, converting an image to a byte array becomes a necessity. This conversion proves highly useful in a variety of scenarios due to the versatility of byte arrays. They offer advantages such as easy comparison, compression, storage, and effortless conversion to other data types. There exist several methods to perform this conversion, but in this context, we present the two fastest and most memory-efficient approaches to achieve this task.
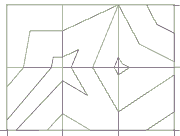
Converting images to byte arrays, developers can access and manipulate the image data directly in a compact and convenient format. This allows for streamlined processing and transmission of image data, making it suitable for various applications, such as image compression, data storage, and transmission over networks. Implementing the fastest and memory-efficient conversion methods ensures optimal performance and resource utilization, ultimately leading to enhanced overall efficiency in handling image data.
Using MemoryStream
The Image object in the .NET Framework provides a convenient save function that enables developers to preserve an image to a file in various supported image formats. In this context, the save function is applied to the MemoryStream object, with the option to specify an image format. Since the MemoryStream object resides in memory, it facilitates easy conversion into a byte array using the ToArray function, which is a method available to the MemoryStream object.
This approach proves advantageous when there's a need to process or transmit image data without physically writing it to a file. By utilizing the MemoryStream object, developers can effectively manipulate and store image data in memory, without the overhead of disk operations. The subsequent conversion to a byte array using the ToArray function further simplifies the handling of image data, allowing for effortless comparison, compression, or transmission to other data types or storage mechanisms.
C#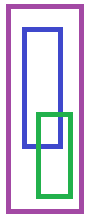
It is essential to handle resource cleanup properly to avoid potential memory leaks when working with MemoryStream and Image objects. Both these objects implement the IDisposable interface, and therefore, it is crucial to dispose of them correctly.
To ensure proper disposal and prevent memory leaks, it is recommended to use the "using" statement, which automatically calls the Dispose() method on objects implementing IDisposable after the block is executed, even if an exception occurs.
Additionally, we can make use of the RawFormat property of the Image object, which provides information about the file format of the image. This property returns an ImageFormat object that can be used to specify the image format when saving the image to a file.
Using ImageConverter
ImageConverter class can be used to convert Image objects from one data type to another.
C#
VB.Net
Convert ByteArray to Image
C#
VB.Net
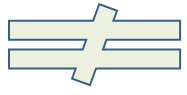
The following program first convert an Image to ByteArray and then convert that byteArray to Image and loads in a picture box.
Source Code | C#
Source Code | Vb.Net
Conclusion
Converting an image to a byte array in C# involves utilizing the Image object's Save function on a MemoryStream and then using the ToArray method from the MemoryStream object to obtain the byte array, allowing for efficient handling and manipulation of image data.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#