MemoryStream to file
The MemoryStream is a powerful mechanism that facilitates the creation of a stream backed by memory, enabling the representation of a pure and seamless in-memory flow of data. In essence, this stream elegantly manages data directly in the computer's memory, bypassing the need for intermediate storage on disk or network access. The utilization of memory as the backing store bestows significant advantages, primarily the remarkable speed it offers in contrast to the comparatively slower disk or network accesses.
MemoryStream
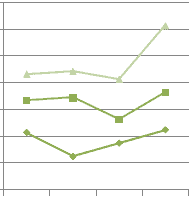
By using the MemoryStream, developers can efficiently handle data without the overhead of writing to or retrieving from physical storage, resulting in improved performance and responsiveness. This proves particularly advantageous when dealing with temporary data, in-memory data manipulation, or situations where rapid data access is essential. The seamless in-memory stream provided by the MemoryStream contributes to a more streamlined and efficient data processing experience, making it a valuable tool in a programmer's arsenal.
The following section explains :
# MemoryStream to File # MemoryStream to StringMemoryStream to FileStream
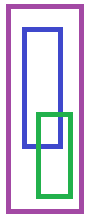
The MemoryStream provides a powerful means to manipulate the data stored in memory directly through a byte array (byte[]), eliminating the need for interaction with files or external resources. The choice of utilizing a byte[] in this context proves highly advantageous due to its fixed size, which simplifies memory allocation and cleanup processes. Moreover, the byte[] incurs minimal overhead, primarily because it allows for direct access to the data without requiring the use of MemoryStream functions.
Save Stream to File
C# MemoryStream to a file
Using the byte[] in conjunction with MemoryStream, developers can efficiently handle and process data solely within the memory space, avoiding the complexities and latencies associated with file I/O or network operations. The byte array's fixed size ensures a more predictable memory management, facilitating better control over resource utilization and enabling swift cleanup when the data is no longer needed.
Save and load MemoryStream to a file
VB.Net MemoryStream to a fileSave MemoryStream to a String
The absence of the need to invoke MemoryStream functions for data manipulation results in a more lightweight and efficient implementation. This streamlined approach proves particularly beneficial when dealing with performance-critical applications or situations requiring frequent data access and modification. The following program shows how to Read from memorystream to a string. Steps follows..
This string is currently saved in the StreamWriters buffer. Flushing the stream will force the string whose backing store is memory (MemoryStream).
How do you get a string from a MemoryStreamNext step is to read this string from memorystream.
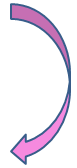
The StreamReader is designed to read data from the current position of the MemoryStream. However, after writing data to the MemoryStream, the current position is set to the end of the string that was previously written. In order to read from the beginning of the data, it is necessary to reset the position of the MemoryStream to the start.
Setting the position to 0, the StreamReader will start reading from the beginning of the MemoryStream, allowing you to access and process the data that was previously written to it. This step is crucial to ensure that you read the data from the desired starting point and avoid missing any information due to the current position being at the end of the string.
Save MemoryStream to a String - C#
Save MemoryStream to a String - VB.Net
There is another methos also help us to read from Memory stream.
Encoding.ASCII.GetString(ms.ToArray());
C# Source CodeConclusion
The combination of MemoryStream and byte[] empowers developers to perform data operations directly in memory, sidestepping the overhead and potential bottlenecks of external resource interactions. This approach not only enhances data processing speed and efficiency but also offers greater control over memory usage and resource management, making it an optimal choice for many memory-bound scenarios.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#