C# DateTime to null
Is it possible to set datetime object to null in C#?
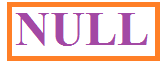
No, because DateTime is a value type
DateTime is a Value Type like int, double etc. so there is no way to assigned a null value.
if(date == null) //WRONG
Value Type and Reference Type
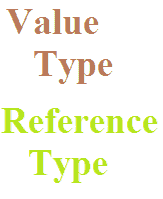
A Value Type holds the data within its own memory allocation and a Reference Type contains a pointer to another memory location that holds the real data.
int num = 100;
Here int is a Value Type and it is stored in an area of memory called the stack.
int[] myArray = new int[100];
Here the space required for the 100 integers that make up the array is allocated on the heap.
How to set DateTime to null in C#
You can solve this problem in two ways, either make it a nullable type, or use the System.DateTime.MinValue.
C# Nullable Type
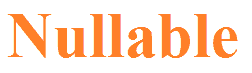
When a type can be assigned null is called nullable, that means the type has no value. All Reference Types are nullable by default, e.g. String, and all ValueTypes are not, e.g. Int32. The Nullable < T > structure is using a value type as a nullable type.
By default DateTime is not nullable because it is a Value Type, using the nullable operator introduced in C# 2, you can achieve this.
Using a question mark (?) after the type or using the generic style Nullable.
Nullable < DateTime > nullDateTime;
or
DateTime? nullDateTime = null;C# Source Code
using System;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Nullable
nullDateTime; //DateTime? nullDateTime = null;
nullDateTime = DateTime.Now;
if (nullDateTime != null)
{
MessageBox.Show(nullDateTime.Value.ToString());
}
}
}
}
Convert String to DateTime
In .Net, you can work with date and time easy with the DateTime class. You can use the methods like Convert.ToDateTime(String), DateTime.Parse() and DateTime.ParseExact() methods for converting a string-based date to a System.DateTime object. More about..... String to DateTime
DateTimePicker Control
The DateTimePicker control has two parts, a label that displays the selected date and a popup calendar that allows users to select a new date. The most important property of the DateTimePicker is the Value property, which holds the selected date and time. More about..... DateTimePicker
How to find date difference ?
A calculation using a DateTime structure, such as Add or Subtract, does not modify the value of the structure. Instead, the calculation returns a new DateTime structure whose value is the result of the calculation. The DateTime.Substract method may be used in order to find the date-time difference between two instances of the DateTime method. More about..... Find date difference
- What is the root class in .Net
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#