Remove spaces from string | C#
A string is a sequence of Unicode characters used to represent text in C#. As mentioned, strings in C# are immutable, meaning they cannot be changed after they are created. However, there are various methods available in C# to manipulate strings and remove specific characters like spaces, newlines, tabs, digits, etc. Let's explore some common string manipulation techniques for removing such characters:
Removing Spaces
To remove spaces from a string, you can use the String.Replace() method:
Removing Newlines
To remove newline characters from a string, you can use String.Replace() as well:
Removing Tabs
Similar to spaces and newlines, tabs can also be removed using String.Replace():
Removing Digits
To remove digits from a string, you can use LINQ and Char.IsDigit() method:
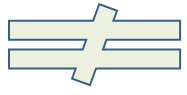
In this example, the LINQ expression filters out all characters that are digits using Char.IsDigit() and creates a new string from the filtered characters.
Depending on the complexity of your requirements, you may need to use regular expressions or other string manipulation techniques. Remember that since strings are immutable, these operations will always create a new string rather than modifying the original one.
Conclusion
Always be cautious when manipulating strings, especially if you are performing multiple operations, as creating new strings repeatedly can have performance implications, especially for large strings or in performance-critical scenarios. In such cases, using StringBuilder can be more efficient, as it allows you to modify the string in place without creating intermediate string objects.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#