Aautoscroll multiline TextBox
TextBox Control
A TextBox control serves a dual purpose in applications, enabling the presentation and acceptance of a single line of text. This versatile control offers additional functionalities beyond the standard Windows text box, elevating its utility to greater heights. Alongside its core capabilities, the TextBox control excels in accommodating multiline editing, allowing users to interact with and modify text across multiple lines seamlessly. Furthermore, it empowers users to safeguard sensitive information with password character masking, a feature that enhances data privacy and security during input operations. The TextBox control's ability to cater to diverse text manipulation scenarios makes it an indispensable asset in creating intuitive and feature-rich user interfaces.
Multiline Text Box
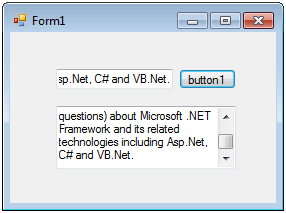
A multiline text box in a graphical user interface facilitates the presentation of multiple lines of text within a single control, expanding its versatility and usability. The Multiline property plays a crucial role in this functionality, as it allows developers to determine whether the TextBox control supports multiline input or restricts it to a single line. By toggling the value of the Multiline property, developers can dynamically configure the behavior of the TextBox control, providing a flexible user interface that adapts to specific text input requirements. This powerful property empowers designers to craft intuitive and interactive forms, enhancing user experiences and enabling efficient manipulation of text across various lines within the same control.
Scroll Bars in Multiline Text Box
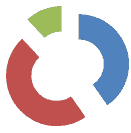
By using the ScrollBars property, developers can seamlessly augment a text box with scroll bars, both horizontally and/or vertically, thereby facilitating an intuitive user experience. This valuable addition empowers users to navigate and explore text content that surpasses the confines of the control's dimensions. With the presence of scroll bars, users can effortlessly view and interact with extended text, ensuring that no valuable information is obscured or lost. By judiciously configuring the ScrollBars property, designers can provide a responsive and user-friendly interface, allowing users to easily traverse through the text and access every bit of crucial information. The inclusion of scroll bars enhances the usability and functionality of the text box, making it a powerful tool for handling diverse text scenarios with utmost ease and convenience.
If you want both scroll bars(vertical and horizondal) you should set ..
If you use TextBox.AppendText(string text), it will automatically scroll to the end of the newly appended text. It avoids the flickering scrollbar if you are calling it in a loop.
Internally, AppendText does something like this:
You can perform another way to achieve scrollbar at the end of the multiline textbox to auto scroll on the TextChanged event:
C#- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#