String and System.string
In C#, string and String both represent the same data type, which is used to store a sequence of characters. The main difference between them lies in their declaration and usage.
string (with a lowercase 's')
- string is an alias for the .NET framework's System.String data type.
- It is a reference type, meaning it is stored on the managed heap, and its variable holds a reference to the actual memory location where the string data resides.
- In C#, it is conventionally recommended to use string (lowercase) instead of String (uppercase).
string firstName = "Smith";
string lastName = "Warrier";
String (with an uppercase 'S')
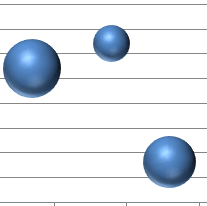
- String is the fully qualified name of the .NET framework's System.String data type.
- It is also a reference type and used to represent a sequence of characters in the same way as string.
String message = "Hello, World!";
As you can see, there is no practical difference between using string and String in C#. Both are interchangeable, and developers typically prefer using string due to its lowercase convention, which aligns with the general naming guidelines in C#.
Here's a more comprehensive example illustrating the interchangeable usage of string and String:
using System;
class Program
{
static void Main()
{
// Using 'string' (lowercase) to declare variables
string name1 = "Alice";
string name2 = "Bob";
// Using 'String' (uppercase) to declare variables
String greeting1 = "Hello, " + name1;
String greeting2 = "Hello, " + name2;
// Using 'string' in method parameters and return type
string fullName = ConcatenateNames(name1, name2);
Console.WriteLine(fullName); // Output: AliceBob
// Using 'String' in method parameters and return type
String fullGreeting = GenerateGreeting(greeting1, greeting2);
Console.WriteLine(fullGreeting); // Output: Hello, AliceHello, Bob
}
static string ConcatenateNames(string firstName, string lastName)
{
return firstName + lastName;
}
static String GenerateGreeting(String greeting1, String greeting2)
{
return greeting1 + greeting2;
}
}
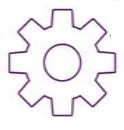
Conclusion
It's important to emphasize that both string and String are interchangeable, and developers generally use string due to the standard naming conventions in C#.
Related Topics
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#