Parse XML - XmlReader
XML is a self-descriptive language, meaning it not only holds the data it contains but also provides the rules and structure to extract that data effectively. When we parse or read an XML file, we are essentially accessing and extracting the information embedded within XML tags present in the file. There are various methods to parse or read an XML document, and this chapter presents some of the simplest and most straightforward approaches to accomplish this task.
XML Parser in C# and VB.Net
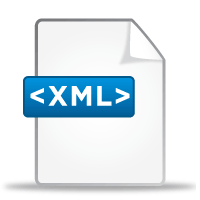
Parsing an XML file, developers can access and utilize the valuable data enclosed within the XML tags, adhering to the defined rules and structure specified within the XML document. The parsing process enables applications to extract and process relevant data, thus facilitating seamless integration and utilization of information stored in XML files.
Throughout this chapter, readers will encounter a range of user-friendly techniques and methods for reading XML files, ensuring ease of implementation and quick understanding. By employing these accessible approaches, developers can effectively interact with XML data, making it a valuable resource for various software applications and data management tasks.
Parse XML with XmlReader
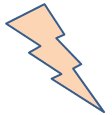
This program demonstrates the implementation of the XmlReader class to efficiently parse an XML string. The XmlReader serves as a superior option, boasting faster processing speeds and lower memory consumption. It enables developers to traverse the XML string methodically, processing one element at a time, and granting access to the element's value before seamlessly advancing to the subsequent XML element. This approach proves particularly advantageous when dealing with large XML documents or scenarios requiring efficient and resource-conscious data extraction and analysis.
C# XmlReader example
Sample XML stringRead XML with XmlReader in C#
C# XML Parser
Output: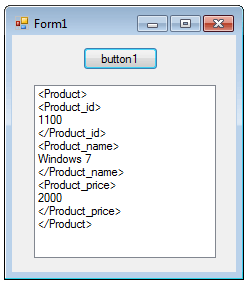
Parse XML with XmlReader in VB.Net
This following program describes how to use the XmlReader class to parse an XML string in VB.Net.
VB.Net XmlReader example
Sample XML stringRead XML with XmlReader in VB.Net
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#