C# Font Dialog Box
Font dialog box represents a common dialog box that displays a list of fonts that are currently installed on the system. The Font dialog box lets the user choose attributes for a logical font, such as font family and associated font style, point size, effects , and a script .
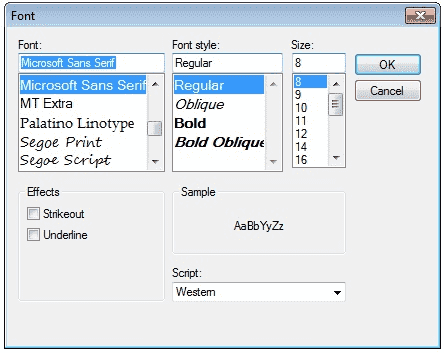
The following C# program invites a Font Dialog Box and retrieve the selected Font Name and Font Size.
Full Source C#
using System;
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
FontDialog dlg = new FontDialog();
dlg.ShowDialog();
if (dlg.ShowDialog() == DialogResult.OK)
{
string fontName;
float fontSize;
fontName = dlg.Font.Name;
fontSize = dlg.Font.Size;
MessageBox.Show(fontName + " " + fontSize );
}
}
}
}
Related Topics
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control