C# Print Dialog Box

A user can use the Print dialog box to select a printer, configure it, and perform a print job. Print dialog boxes provide an easy way to implement Print and Print Setup dialog boxes in a manner consistent with Windows standards.
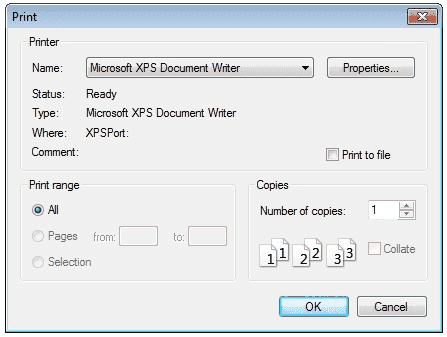
The Print dialog box includes a Print Range group of radio buttons that indicate whether the user wants to print all pages , a range of pages, or only the selected text. The dialog box includes an edit control in which the user can type the number of copies to print . By default, the Print dialog box initially displays information about the current default printer.
How to print a Document in C#?

PrintDocument object represents a document to be printed. It encapsulates all the information needed to print a page. They associate with the control which content can be print. They handle the events and operations of printing. Once a PrintDocument is created, we can set the Document property of PrintDialog as this document. After that we can also set other properties.
How to Format PrintDocument?
If you want to set the size of the paper:
How to Preview PrintDocument
With the help of PrintPreviewDialog You can preview a document also.
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control