Reflection In .NET
.NET Reflection is a powerful feature of the .NET framework that enables developers to inspect and manipulate types, members, and metadata of assemblies at runtime. It provides a way to obtain information about the structure and behavior of classes, interfaces, fields, properties, methods, and other entities within a compiled assembly.
Reflection allows developers to dynamically analyze and interact with code elements, even if their details are unknown at compile time. This is particularly useful in scenarios such as plugin systems, object serialization, dependency injection frameworks, and dynamic code generation.
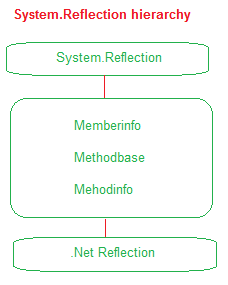
By utilizing reflection, developers can perform various tasks such as:
Obtaining Type Information
Reflection enables developers to retrieve information about types present in an assembly, including their properties, methods, fields, events, and attributes. This information can be utilized for various purposes, such as creating instances of types dynamically or invoking their members.
Dynamic Code Generation
Reflection allows developers to create types, methods, properties, and other members dynamically at runtime. This can be useful for scenarios where code needs to be generated dynamically based on certain conditions or configurations.
Accessing Attributes
Reflection allows developers to inspect and utilize custom attributes defined on types, methods, properties, and other code elements. This enables the retrieval of additional metadata or behavior associated with these elements.
Modifying and Extending Existing Code
Reflection allows developers to modify existing code at runtime by manipulating the members of types, adding new properties or methods, changing values, or accessing private members that would otherwise be inaccessible.
Conclusion
It's important to note that reflection can have performance implications and should be used sensibly, as it involves runtime introspection and dynamic invocations. However, when used appropriately, reflection can provide great flexibility and extensibility in developing dynamic and adaptable applications within the .NET framework.
- C# Interview Questions (part-1)
- C# Interview Questions (part-2)
- C# Interview Questions (part-3)
- Difference between a Debug and Release build
- Difference between normal DLL and .Net DLL
- What is an Interface in C#
- Difference between Abstract Class and Interface in C#
- Difference between a thread and a process
- Delegates in C# with Examples
- Differences between a control and a component
- Differences between Stack and Heap
- Globalization and Localization | C#
- What is .Net serialization
- Difference between web service and .net remoting
- Difference between managed and unmanaged code
- Difference between Shallow copy and Deep copy
- Use of System.Environment Class
- What is the difference between private and shared assembly?
- Does the .NET have in-built support for serialization?
- How to properly stop the Thread in C#?
- Why are there five tracing levels in System.Diagnostics.TraceSwitcher?
- Why is XmlSerializer so slow?
- How many types of Jit Compilers?