Global variables in Java
In Java, true global variables, as commonly understood in other programming languages, do not exist due to the strict scoping rules. All variables in Java must be declared within the scope of a class, method, or block. Consequently, variables cannot be directly accessible from outside their defined scope, ensuring better encapsulation and data integrity within classes and methods. The absence of traditional global variables promotes a more structured and organized approach to programming, encouraging developers to explicitly define the scope and visibility of their variables, leading to more maintainable and secure code.
Global variable
In programming, a global variable is a variable that is declared at the highest level of scope in a program and is accessible from any part of the program. It is not confined to any specific function or method, allowing it to be accessed and modified from various parts of the codebase. However, the use of global variables should be approached with caution, as they can lead to code complexity, hinder maintainability, and introduce unintended side effects. It is generally recommended to minimize the use of global variables and favor encapsulation and proper data passing techniques for better code organization and reliability.
Global variables can be convenient for storing values that need to be accessed across different functions or methods in a program. However, their use should be carefully considered due to potential drawbacks. Modifying global variables from various parts of the program can introduce unintended side effects and make it challenging to track the flow of data. As a result, it may lead to harder maintenance and debugging processes. It is advisable to limit the use of global variables and opt for more structured approaches, such as passing data explicitly between functions or using object-oriented principles to encapsulate data within classes. This can improve code readability, maintainability, and reduce the risk of unexpected behaviors.
Global Variable in Java
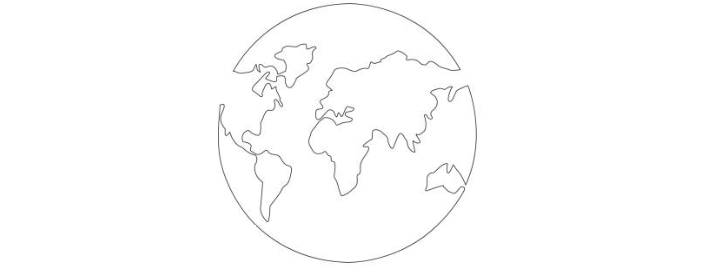
The concept of true global variables, as commonly understood in some other programming languages, is not present because all variables must be declared within a class, method, or block scope. However, Java offers alternatives in the form of "class variables" and "instance variables," which can be accessed by all methods within a class.
Class variables, also known as static variables, are associated with the class itself rather than with specific instances of the class. They retain their values across all instances of the class and can be accessed using the class name. Class variables are declared with the "static" keyword.
Instance variables, on the other hand, are specific to each instance of the class and hold unique values for each object. They are declared without the "static" keyword and belong to the object itself.
Both class variables and instance variables are accessible from any method within the class, providing a form of shared data that can be accessed by multiple methods. However, it is essential to use these variables with care and consider encapsulation and proper access control to maintain the integrity of the data and prevent unintended side effects.
Class variables
Class variables, also known as "static variables" or "static fields," are declared using the static keyword and are associated with the class itself rather than with any specific instance of the class. They retain their values across all instances of the class and are shared by all objects of that class. Class variables can be accessed by any method within the class, as well as by methods in other classes if they are declared public or protected.
Since class variables are shared among all instances of the class, they are useful for storing data that is common to all objects of that class. For example, a class representing a car could have a class variable to keep track of the total number of cars created, as this information is relevant to all instances of the car class.
It's important to note that when modifying the value of a class variable in one instance of the class, the change will be reflected in all other instances, as they all share the same class variable. Therefore, caution should be exercised when using class variables to avoid unintended side effects.
Class variables play a significant role in Java programming as they provide a way to share data among different instances of a class and offer a means to maintain common values and behaviors at the class level. However, proper access control and encapsulation should be considered to ensure data integrity and maintainable code.
Instance variables
Instance variables are declared within the class but outside of any method or static block. Unlike class variables, each object or instance of the class has its own unique copy of instance variables. These variables are associated with the state of individual objects and can hold different values for each object. Instance variables can be accessed and modified by any method within the class, as they are part of the object's state.
Instance variables play a crucial role in defining the properties or characteristics of objects in a class. For example, in a class representing a student, instance variables such as "name," "age," and "grade" would hold unique values for each student object.
Since instance variables are specific to individual objects, changes to one object's instance variables do not affect the instance variables of other objects of the same class. This encapsulation ensures that each object maintains its own state, independent of other objects.
Access to instance variables outside the class is controlled through methods like getters and setters, which provide controlled access to these variables. Public or protected methods can be used to expose instance variables to other classes while maintaining proper access control.
Class and instance variables provide a way to store data that can be shared among different methods within a class or among instances of that class, respectively. However, their scope is limited to the class or object level, promoting encapsulation and reducing the risk of unintended side effects. This makes the code more manageable, easier to maintain, and less prone to bugs caused by global variables.
On the other hand, local variables are declared within a specific method or block and have the smallest scope among the three types of variables. They are only accessible within the method or block in which they are declared, providing a level of isolation that prevents unintended changes to variables from affecting other parts of the program. Using local variables where possible enhances code readability and reduces memory usage as they are automatically released from memory once the method or block finishes execution.
Conclusion
By favoring class, instance, and local variables over global variables, developers can follow best practices in Java programming, achieving better code structure, maintainability, and performance. This approach aligns with the principles of object-oriented programming and helps create robust and efficient applications.