Java Program to Check Leap Year
What is a Leap Year?
The Earth takes approximately 365.25 days to complete one orbit around the Sun, and this is known as a solar year. However, to simplify the calendar, we round the days in a year to 365. To account for the additional fractional day, we add one extra day to the calendar approximately every four years. This results in a leap year, which contains 366 days instead of the usual 365 days. The extra day is inserted in February, making it a month with 29 days instead of the usual 28 days.
How to determine whether a year is a leap year
- If it is evenly divisible by 4
- Unless it is also evenly divisible by 100
- Unless it is also evenly divisible by 400
The year 2000 was a leap year, because it obeys all three rules 1, 2, and 3, and therefore was a leap year.
Algorithm
- If year is not divisible by 4 then it is a common year
- Else if year is not divisible by 100 then it is a leap year
- Else if year is not divisible by 400 then it is a common year
- Else it is a leap year
How to Check Leap Year in Java?
You can write a program to check if a given year is a leap year or not. A leap year is a year that is divisible by 4, except for years that are divisible by 100. However, years divisible by 400 are also considered leap years.
Following is a Java program to check if a year is a leap year:
In this program, we first take input from the user for the year to be checked. Then, we call the isLeapYear() method to determine whether the year is a leap year or not.
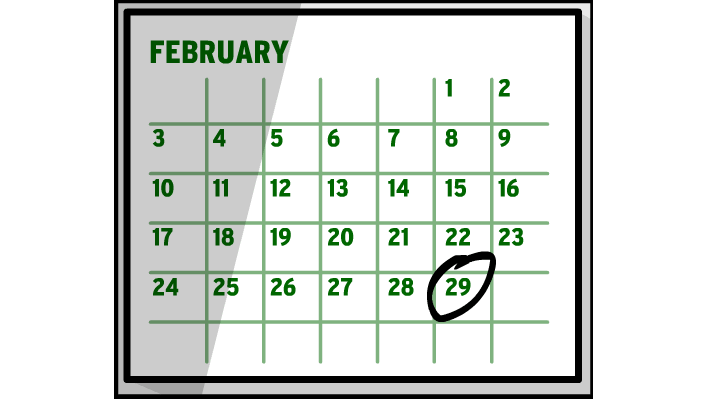
The isLeapYear() method checks the conditions for a leap year using if-else statements. If the year is divisible by 4 and not divisible by 100, or it is divisible by 400, then it returns true, indicating that the year is a leap year. Otherwise, it returns false, indicating that the year is not a leap year.
Conclusion
You can run this program and input different years to check if they are leap years or not.