Can you overload main() method in Java?
Yes, in Java, you can overload the main() method, which means that you can define multiple main() methods in a class with different parameter lists.
What is Method overloading
Method overloading is a powerful feature in Java that enables a class to have multiple methods with identical names but distinct parameter lists. This means you can define multiple methods within a class, as long as their input parameters vary in terms of number, type, or arrangement. Overloading facilitates code reusability and readability by providing more flexible ways to call methods, tailoring them to different data types or quantities of arguments. By utilizing method overloading effectively, Java programmers can create cleaner and more concise code while accommodating diverse scenarios and input requirements.
Overload main() method in Java
Method overloading is a feature in Java that allows a class to have multiple methods with the same name but different parameter lists. When you overload the main() method, you are providing different entry points to your program, each with different input parameters.
Following is an example of overloading the main() method:
In this example, we have three different main() methods. The first one is the standard entry point for Java programs, which takes a String array as an argument. The other two methods are overloaded versions of main(), one taking a String argument and the other taking an int argument.
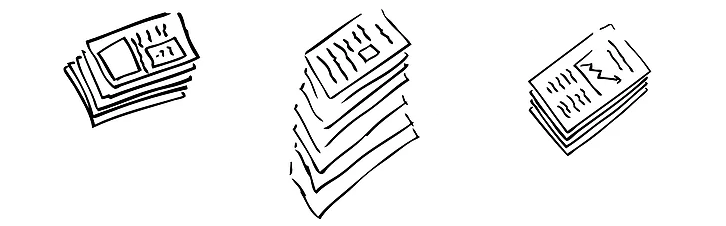
When you run the MainOverloadExample class, Java will call the standard main() method with the String array argument by default. However, you can also call the other main() methods explicitly with appropriate arguments:
Conclusion
Remember that when you run a Java program, you can only specify one entry point on the command line. However, the overloaded main() methods can still be called from within the program as regular methods. They are useful when you want to provide different ways to start the program or handle different types of input arguments.