Uncaught TypeError: 'undefined' is not a function
This prevalent JavaScript error arises when attempting to invoke a function prior to its definition. It transpires when an uninitialized or inadequately initialized function is executed. This error indicates that the expression does not yield a function object, thereby necessitating an evaluation of the context to ascertain that the attempted execution does not involve a function.
Reverse the order
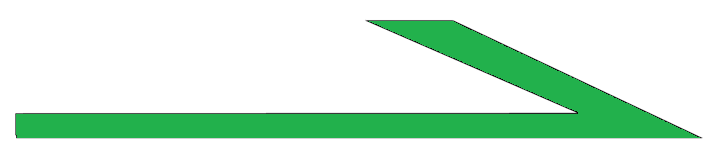
For instance, while this code works as expected:
If you reverse the order of the statements the Uncaught TypeError: undefined is not a function error will occur:
So, It's better you double check that your scripts are being loaded correctly.
This weird behaviour depends on how you define the functions and when you call them.
examplesThis is because of something called hoisting!
JavaScript Function
In Javascript , when you execute a function, it's evaluated like the following:
- expression.that('returns').aFunctionObject(); // js
- execute -> expression.that('returns').aFunctionObject // what the JS engine does
That expression may be complex. So encountering the "undefined is not a function" error implies that the evaluated expression fails to yield a function object. Thus, it becomes crucial to discern that the element under execution is not a function. This situation warrants careful examination of the expression to pinpoint the discrepancy causing the error.
File order (library)
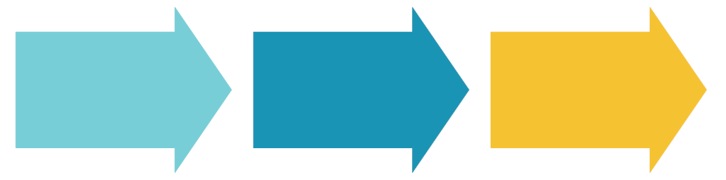
Occasionally, errors can arise due to inadequate or incorrect loading of libraries. In libraries like jQuery, it's imperative to ensure the proper loading of the library before engaging in jQuery functions. This entails correctly incorporating the library into the webpage to facilitate the successful execution of desired jQuery operations. It should be something like:
- jQuery.min.js
- jquery-ui.js
- any third party plugins you loading
- your custom JS
bind() method
This error manifests in Chrome when an attempt is made to call an undefined function. It can be validated by testing the scenario in the Chrome Developer Console and Mozilla Firefox Developer Console, where the error will become evident upon execution. Consider the following example:
When you execute the above code and then click on the page, an error surfaces: "Uncaught TypeError: this.myMessage is not a function." This error arises due to the anonymous function's execution context being bound to the document, while "myMessage" is defined on the window object. Consequently, the function cannot access "myMessage" as it resides in a different scope.
You can fix this issue by using the bind() method to pass the proper reference:
Conclusion
"Uncaught" signifies that the error wasn't captured within a try-catch block. The TypeError object denotes an error arising from a value that doesn't match the anticipated type. Additionally, "Undefined" pertains to a property within the global object. When a variable lacks an assigned value, it falls under the "undefined" type.