Blocked a frame with origin from accessing a cross-origin frame
The Same-Origin Policy (SOP) serves as a security measure that limits the interactions between documents or scripts loaded from one origin and resources from another origin. For instance, when Site X attempts to retrieve content from Site Y within a frame, Site Y's pages are not directly accessible by default due to security concerns. This policy prevents potential security vulnerabilities that could arise if unrestricted access between different origins was allowed.
An origin is categorized as distinct if any of the following components in the address are not consistent:
Protocol, hostname and port must be the same of your domain if you want to access a frame.
How to solve?
The window.postMessage() function offers a secure way to overcome this limitation. It allows controlled cross-origin communication between Window objects, such as between a parent page and an embedded iframe, ensuring enhanced security while facilitating interaction.
- targetOrigin - specifies what the origin of targetWindow must be for the event to be dispatched, either as the literal string "*" (indicating no preference) or as a URI.
example
Main Page source
The second argument of postMessage() can be '' to signify a lack of preference regarding the destination's origin. However, it is recommended to always specify a specific targetOrigin rather than using '' if you are aware of the intended location of the other window's document. Failing to provide a specific targetOrigin can lead to unintended exposure of the transmitted data to potentially malicious sites.
The dispatched event
In your ‹iframe› contained in the main page, a window can listen for dispatched messages by executing the following JavaScript:
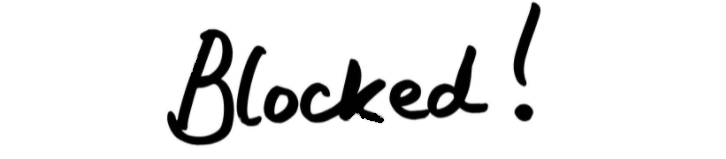
Disabling same-origin policy in your browser
Running a browser with disabled same-origin security settings allows any website to access cross-origin resources without restrictions.
For Windows:
Navigate to the command prompt and access the folder containing Chrome.exe by entering the following command:
For Linux :
Also if you're trying to access local files for development purposes like AJAX or JSON, you can use this flag too.
NOTE: Disabling same-origin policy is very unsafe and should NEVER be done if you do not know exactly what you are doing.
Conclusion
The message "Blocked a frame with origin from accessing a cross-origin frame" indicates that a frame originating from one domain was prevented from accessing a frame from a different domain due to the same-origin policy. This policy aims to enhance security by restricting interactions between frames from distinct origins.