XMLHttpRequest cannot load. Origin is not allowed by Access-Control-Allow-Origin
This error occurs due to the CORS (Cross-Origin Resource Sharing) error. A Cross-Origin Request refers to a resource request, such as an image or font, originating from a different location. CORS regulates these cross-origin requests. For instance, while writing HTML and JavaScript in a local code editor and testing it in a browser, you may encounter error messages concerning Cross-Origin Requests. Notably, issuing requests through XMLHttpRequest to other domains or subdomains is restricted.
JSONP (JSON with Padding)
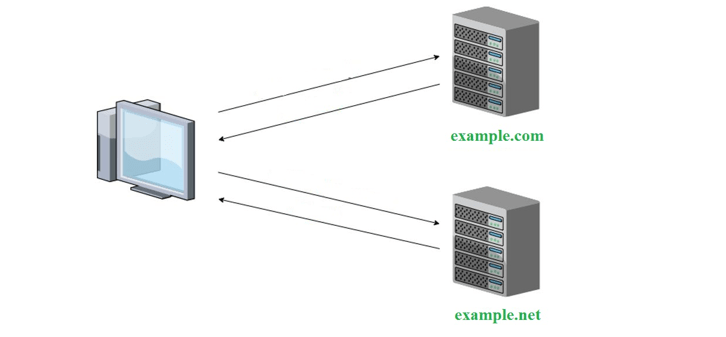
JSONP (JSON with Padding) serves as a widely employed technique for circumventing the cross-domain limitations enforced by web browsers. In a scenario where you're situated on the domain example.com but require a request to domain example.net, the need to navigate across domain boundaries arises. JSONP offers a straightforward workaround to surmount the same domain policy that constrains XMLHttpRequest. This method entails replacing XMLHttpRequest with <script> HTML tags—typically reserved for loading JavaScript files—enabling JavaScript to retrieve data from an alternate domain.
JSONP requests diverge from the conventional XMLHTTPRequest and related browser mechanisms. Instead, they involve crafting a <script> tag with its source directed toward the intended URL. This constructed script tag is subsequently injected into the DOM, usually within the <head> element. This distinctive approach enables the retrieval of data by using the script loading mechanism, facilitating cross-domain data exchange without running afoul of the same-origin policy.
JSONP Request:
Here you can see the JSONP response object is passed as an argument to a callback function.
Hence, JSONP requests incorporate the inclusion of a callback parameter. This parameter communicates the designated function's name to the server, which employs this function to envelop the response. It is imperative that this function is present within the global scope when the <script> tag is executed by the browser upon request completion. In this context, consider a response object as an example: { "bar" : "baz" }.
That's all there is to know about JSONP : it's a callback and script tags.

JSONP (JSON with Padding) functions by appending a <script> element to the document to fetch data in the form of a JavaScript program. This program then invokes an existing function within the page. However, employing the JSONP approach on a URL that delivers JSON data, as opposed to JavaScript, will result in failure, often leading to a CORS error. This disparity arises from the distinction between JSON and JavaScript data structures, rendering JSONP incompatible with URLs returning JSON content.
Local Development (Chrome)
In straightforward terms, this error emerges when an attempt is made to access a domain or resource from a different domain. If you encounter this issue during local development and are utilizing Chrome as your browser, you can mitigate it by modifying Chrome's security settings through the addition of specific command-line arguments, as demonstrated below:
Localhost
To enable Cross-Origin Resource Sharing (CORS) for localhost on the server, you must include the following configuration in the request header:
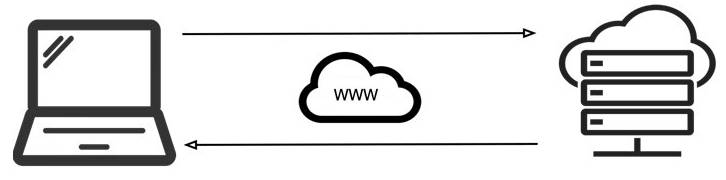
Using Proxy
Another convenient approach is to establish a proxy on your local server. This proxy would intercept the remote request, and subsequently relay it back to your JavaScript code. This strategy effectively bypasses the CORS restrictions by channeling the requests through your local server.
Browser extensions
Cross-Origin Resource Sharing (CORS) is inherently restricted in contemporary browsers through default settings in JavaScript APIs. However, the capability exists for a browser extension to introduce CORS headers into responses prior to the enforcement of the Same Origin Policy (SOP). Although this can be advantageous during development stages, it's crucial to recognize that such a method isn't a viable solution for production websites due to its impracticality and potential security implications.
Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) functions as a deliberate mechanism for sharing data or resources from a website with a third-party site as required. This involves the utilization of supplementary HTTP headers to instruct browsers to permit a web application hosted on one origin to access specific resources from a distinct origin. CORS serves as an extension to and enhances the versatility of the same-origin policy (SOP). It's important to note that while CORS offers valuable benefits, if a website's CORS policy is inadequately configured or implemented, it can also introduce potential vulnerabilities and susceptibility to cross-domain attacks.
Same-Origin Policy (SOP)
The Same-Origin Policy (SOP) holds a major role as a vital security mechanism, confining the extent to which a document or script originating from one source can engage with resources from another origin. Browsers facilitate the simultaneous loading and presentation of resources from numerous websites, whether through multiple open tabs or embedded iframes from diverse sites. In the absence of restrictions on interactions among these resources, a compromised script could lead to unauthorized exposure of a user's browser content. The SOP safeguards against this by obstructing read access to resources sourced from dissimilar origins.
Supported by virtually all contemporary browsers, the Same-Origin Policy (SOP) stands as a foundational rule upheld by web browsers, orchestrating the flow of data between distinct websites and web applications. An origin's definition hinges on the URL's scheme, host, and port components. This browser security feature operates as a regulatory framework, governing the interplay between documents and scripts from one origin and resources from another. The SOP is instrumental in averting the undue access to the Document Object Model (DOM) of unrelated pages, which could otherwise result in the retrieval of sensitive data and the execution of actions across different web pages without user authorization.
Conclusion
The error message "XMLHttpRequest cannot load. Origin is not allowed by Access-Control-Allow-Origin" signifies that a request made through XMLHttpRequest is being blocked due to the lack of Access-Control-Allow-Origin header in the response. This error arises when the same-origin policy is violated, preventing cross-origin requests without the necessary CORS headers in place.