Access Mode of Files | Python
File access mode is a string that specifies the mode in which a file should be opened. In Python, when you open a file using the open function, you can specify the mode in which the file should be opened. There are several file access modes available in Python:
- "r" (read mode): Open the file for reading. If the file does not exist, a FileNotFoundError is raised.
- "w" (write mode): Open the file for writing. If the file exists, its contents are truncated. If the file does not exist in the file path, a new file is created.
- "a" (append mode): Open the file for writing, but instead of truncating its contents, new data is added to the end of the file. If the file does not exist in the file path, a new file is created.
- "x" (exclusive creation mode): Open the file for writing, but only if the file does not already exist. If the file exists, a FileExistsError is raised.
- "b" (binary mode): Open the file in binary mode. When used in combination with other modes, the "b" mode changes the way data is read from or written to the file. For example, "rb" opens the file in binary read mode, and "wb" opens the file in binary write mode.
- 't': Text mode: Used in combination with other modes to indicate that the file should be opened in text mode (default).
- "+" (read and write mode): Open the file for reading and writing. The file's pointer is positioned at the starting of the file.
- 'r+' Read and write mode: Opens the file for reading and writing and the pointer is placed at the beginning of the file. If the file does not exist, an error is raised.
- 'a+' Read and append mode: Opens the file for reading and writing and the pointer is placed at the end of the file. If the file does not exist in the file path, it is created.
Python File Access Mode | examples
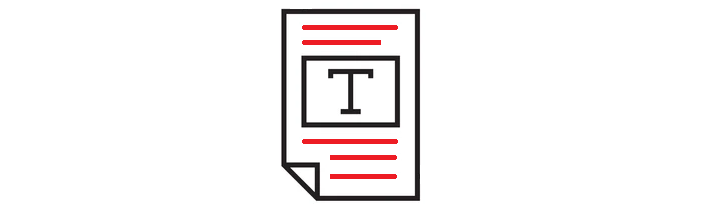
Here are some examples of using the different file access modes in Python:
'r' (Read-only mode):
with open('file.txt', 'r') as f: # open the file in read-only mode.
content = f.read()
print(content)
'r+' (Read and write mode):
with open('file.txt', 'r+') as f: # open the file in read and write mode
content = f.read()
print(content)
f.seek(0) # Seek to the beginning of the file
f.write('This is a new line.') # Write to the file
'w' (write mode):
with open("file.txt", "w") as f: # Open the file in write mode
f.write("Hello, world!") # Write "Hello, world!" to the file
'a' (Append mode):
with open('file.txt', 'a') as f: # Open the file in append mode
f.write('This is a new line.') # Append to already existing content
'a+' (Read and append mode):
with open('file.txt', 'a+') as f: # Open the file in read and append mode
content = f.read()
print(content)
f.seek(0, 2) # Seek to the end of the file
f.write('This is a new line.') # Append to the file
'b' (binary mode):
with open("file.bin", "wb") as f: # Write binary data to a file
f.write(b"\x01\x02\x03\x04")
with open("file.bin", "rb") as f: # Read binary data from a file
data = f.read()
print(data)
//Output:# b'\x01\x02\x03\x04'
Conclusion
File access mode in Python defines the manner in which a file is opened and manipulated. It specifies whether the file is to be read, written, appended to, or a combination of these operations. Common modes include "r" for reading, "w" for writing (creating or overwriting), and "a" for appending to an existing file.
Related Topics