seek() and tell() methods in Python
The seek and tell methods in Python are used for manipulating the current position of the file pointer in a file.
seek() method
The seek method allows you to move the file pointer to a specific position in the file. You can use it to reposition the file pointer to a specific location, so that subsequent reads or writes will start from that position. Syntax:
The seek method takes two arguments: the first is the offset from the beginning of the file, and the second is an optional reference point that specifies where the offset is relative to. By default, the reference point is the beginning of the file. The offset can be positive (to move the pointer forward) or negative (to move the pointer backward).
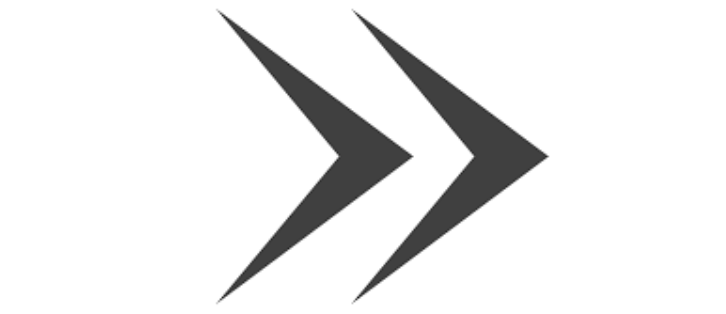
tell() method
The tell method returns the current position of the file pointer, in bytes from the start of the file. The position is an integer that represents the number of bytes from the beginning of the file. Syntax:
You can use it to determine the current position of the file pointer, for example, to keep track of how much of a file has been processed.
Note that when you open a file in text mode, the tell method returns the number of characters, not the number of bytes. In binary mode, the tell method returns the number of bytes.
Conclusion
The seek() and tell() methods are used for file cursor manipulation. The seek() method repositions the cursor to a specified byte offset, facilitating navigation within the file, while the tell() method returns the current cursor position. These methods aid in precise file navigation and data manipulation.