How to use Split in Python
The split() method in Python operates by partitioning a string or line into distinct words, utilizing a designated delimiter string as the basis for segmentation. This method yields a collection of one or more novel strings, each of which corresponds to a distinct substring created by the segmentation process. As a result, the substrings are systematically collated and presented within the context of a list datatype, facilitating efficient data organization and manipulation.
Syntax- Separator:The is a delimiter. The string splits at this specified separator. If is not provided then any white space is a separator.
- Maxsplit: It is a number, which tells us to split the string into maximum of provided number of times. If it is not provided then there is no limit.
- Return:The split() breaks the string at the separator and returns a list of strings.
In the absence of a explicitly defined separator when invoking the function, the default behavior of the split() function in Python involves utilizing whitespace as the delimiter. In more straightforward terms, the separator signifies a predetermined character that functions as an intermediary between individual variables during the segmentation process. The functionality of the split() function when provided with an empty string hinges on the value assigned to the "sep" parameter. If "sep" is unspecified or set to None, the outcome will be an empty list. Conversely, if "sep" is specified as any string value, the result will consist of a list housing a single element, which is an empty string.
Splitting String by space
The split() method functions without a specified argument, leading to segmentation based on whitespace.
exampleSplitting on first occurrence
In the following example, it will Split by first 2 whitespace only.
exampleSplitting lines from a text file in Python
The following Python program reading a text file and splitting it into single words in python
exampleSplitting String by newline(\n)
Splitting String by tab(\t)
Splitting String by comma(,)
Split string with multiple delimiters
In this case Python uses Regular Expression.
exampleSplit a string into a list
The following Python program split a string to a List.
examplemaxsplit parameter
Split the string into a list with max 2 items
In the above program maxsplit is 2, the first two string are split and rest of them are in a same string.
Split a string into array of characters
Python split() using substring
Extact a string after a specific substring.
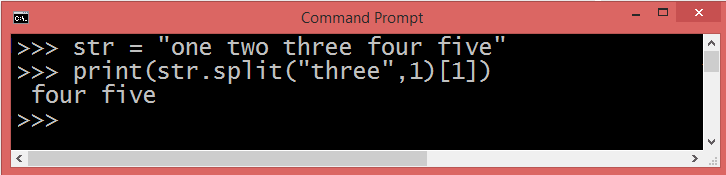
In the above example, you can see the split() function return next part of a string using a specific substring.
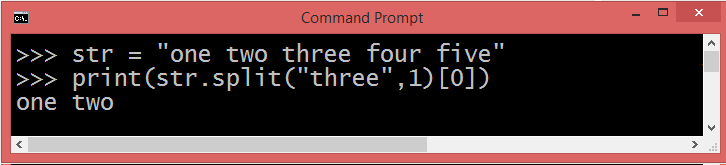
Here, you can see the split() function return the previous part of the string using a specific substring.
Conclusion
The split() method is employed to divide a string into distinct segments based on a designated separator. By default, if no separator is specified, whitespace is used as the separator. This method facilitates effective string segmentation, aiding in data processing and manipulation.