Python Break and Continue statement
Python break statement
In certain situations, it becomes necessary to omit specific statements within a loop or abruptly terminate the loop without evaluating the test expression. To address this, Python provides the "break" statement. This statement enables an immediate exit from the loop, circumventing its regular termination condition and allowing for more controlled program flow.
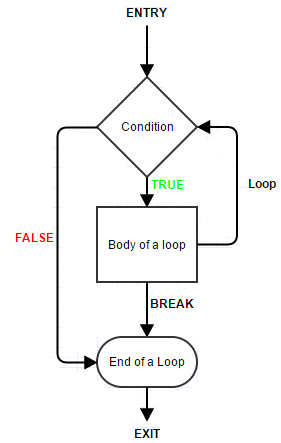
As seen in the above image, upon encountering the "break" statement within a loop, the loop is promptly concluded, and program control transitions to the subsequent statement following the loop. This mechanism facilitates an abrupt exit from the loop's iteration.
break statement in while loop
In the above program, when n==5, the break statement executed and immediately terminated the while loop and the program control resumes at the next statement.
break statement in while loop
Python continue statement
The "continue" statement in Python operates similarly to "break," albeit with a distinct focus. While "break" enforces the termination of the loop, "continue" triggers the immediate initiation of the next iteration of the loop, effectively bypassing the remaining code within the loop body.
continue statement in while loop
In the above program, we can see in the output the 3 is missing. It is because when n==3 the loop encounter the continue statement and control go back to start of the loop.
continue statement in for loop
In the above program, we can see in the output the 3 is missing. It is because when n==3 the loop encounter the continue statement and control go back to start of the loop.
Conclusion
Python's "break" statement provides the means to promptly exit a loop, while the "continue" statement allows for the immediate progression to the subsequent iteration of the loop, bypassing the remaining code in the current iteration. Both statements contribute to controlling loop execution and tailoring program flow.